Disable _draw in Godot
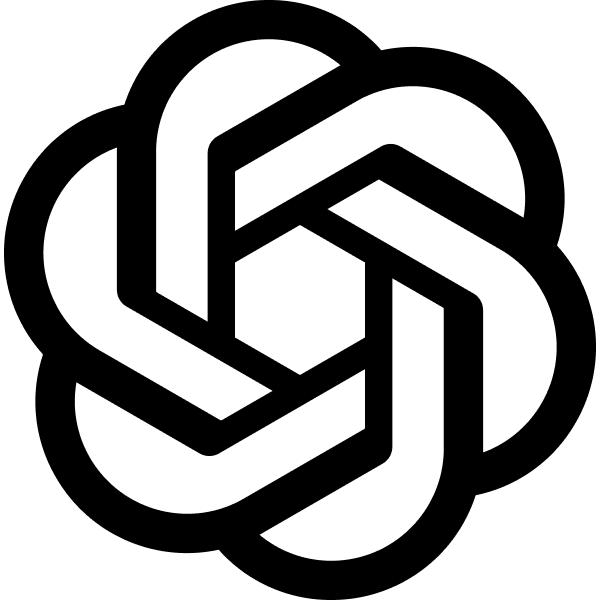
TODO: collapasable, copyable, download, etc
In Godot 4, I attached a script to an object and implemented _draw
. How is this easily disabled?In Godot 4, if you want to disable the _draw
function, you can use the update()
method conditionally and control whether the custom drawing should happen or not. Here's a basic approach to achieve this:
- Using a flag to control drawing:
Define a boolean flag that determines whether drawing should occur. Based on this flag, you can conditionally call the
update()
method which triggers the_draw
function.
extends Node2D
var draw_enabled = false
func _ready():
# Initial state: drawing is disabled
draw_enabled = false
func _process(delta):
if Input.is_action_just_pressed("ui_toggle_draw"):
draw_enabled = !draw_enabled
update() # Trigger a redraw
func _draw():
if not draw_enabled:
return
# Your drawing code here
draw_circle(Vector2(50, 50), 30, Color.red)
In this example:
draw_enabled
controls whether the_draw
function should execute its drawing code.ui_toggle_draw
is an input action defined in the Input Map (e.g., toggling drawing with a key press).- When the
draw_enabled
flag is toggled,update()
is called to request a redraw, and_draw
will execute based on the flag's state.
- Disable drawing by not calling
update()
: Another approach is to control when theupdate()
method is called, which determines when_draw
is executed. If you never callupdate()
,_draw
will not be executed.
extends Node2D
var draw_enabled = false
func _ready():
# Initial state: drawing is disabled
draw_enabled = false
func _process(delta):
if Input.is_action_just_pressed("ui_toggle_draw"):
draw_enabled = !draw_enabled
if draw_enabled:
update() # Trigger a redraw if drawing is enabled
func _draw():
# Your drawing code here
draw_circle(Vector2(50, 50), 30, Color.red)
In this example:
- The
_draw
function will only be called ifdraw_enabled
is true andupdate()
is explicitly called.
Choose the approach that best fits your use case. The first approach gives you finer control within the _draw
function, while the second approach manages the enabling and disabling by controlling the update()
calls.
Related Chats
Client-Only Component in SvelteKit 0.345
Reset Saving State Strategically 0.332
Draw Rects & Ovals 0.304
Tests untuk initializeNewThread 0.293
Cleaned-Up Svelte Code 0.292
Svelte: Force New Component 0.287
Svelte Component Help 0.287
Server-Side Code Placement 0.282
Wrap in useCallback Hook 0.278