Lightsaber Trainer App
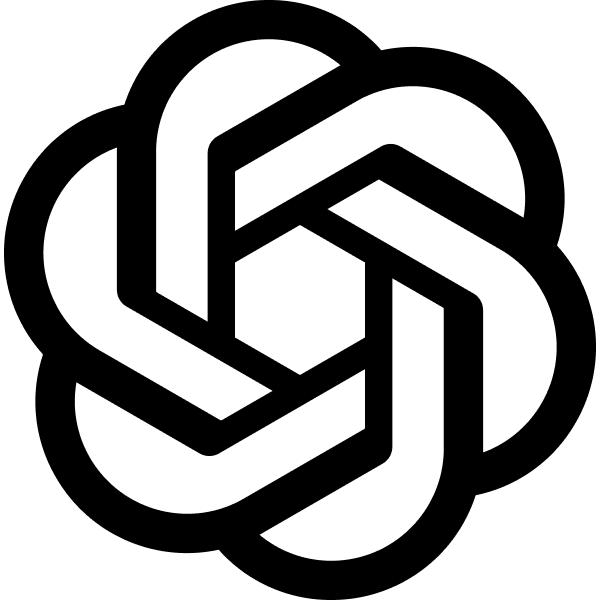
TODO: collapasable, copyable, download, etc
I need an iPhone app that has the following Welcome screen. An image at the top. The image should be sized based on the width to take up the full width ignoreing unsafe edges. Next it should have a title "Lightsaber Trainer" in the center. Next It should have a text area. Lastly, it should have a button "Get Started". The image at the top and the button at the bottom and the rest of the content should be spaced to fill the middle nicely.
When you click get started, it loads a PreparingView Spatial computing goes even further with visionOS updates that bring enhanced support for volumetric apps, powerful new enterprise APIs, the new TabletopKit framework, and more.
visionOS Highlights
TabletopKit Create collaborative experiences centered around a table. This new framework handles the manipulation of cards and pieces, establishing placement and layout, and defining game boards.
New enterprise APIs provide access to spatial barcode scanning, the Apple Neural Engine, object tracking parameters, and more.
visionOS for enterprise
Volumetric APIs • Create apps that take full advantage of depth and space — and can
run side by side with other apps in the Shared Space.
• Resize volumes by using the SwiftUI scene
modifier windowResizability.
Decide if you want the user’s hands to appear in front of or behind your digital content.
Inputs
Getting started? Explore the visionOS Pathway on developer.apple.com
• Detect planes in all orientations.
• Allow anchoring objects on surfaces in your surroundings.
• Use Room Anchors to consider the user’s surroundings on a
per-room basis.
• Attach content to individual objects around the user with the new
Object Tracking API for visionOS.
Updates to scene understanding
To create an iPhone app with the specified Welcome screen and navigation functionality, you can use SwiftUI for a clean and concise implementation. Below is a code example to achieve the desired layout:
- WelcomeView: The main welcome screen with an image, title, text area, and button.
- PreparingView: The view to be loaded when the "Get Started" button is pressed.
import SwiftUI
struct WelcomeView: View {
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
struct PreparingView: View {
var body: some View {
VStack {
Text("Preparing your experience...")
.font(.title)
.padding()
// Add more content for the PreparingView as needed
}
}
}
@main
struct LightsaberTrainerApp: App {
var body: some Scene {
WindowGroup {
NavigationView {
WelcomeView()
}
}
}
}
Explanation:
WelcomeView:
- A
GeometryReader
is used to make the image take the full width and a specific height proportionate to the width. - The image uses the
resizable()
andscaledToFill()
modifiers to ensure it fills the width while maintaining its aspect ratio. - The
Spacer()
elements are used to distribute the remaining space evenly. - The
Text
elements are styled for the title and description. - A
NavigationLink
is used for the "Get Started" button to navigate to thePreparingView
.
- A
PreparingView:
- A simple view with a text message indicating the preparation state.
App Structure:
- The
LightsaberTrainerApp
struct initializes the app with aNavigationView
to enable navigation between views.
- The
Note:
- Replace
"yourImageName"
with the actual name of your image asset. - You might want to further customize the
PreparingView
to include more relevant content for the preparation phase.I need a snippet of SwiftUI code. Please output a VStack that uses SF Symbols and Labels for the following information:
- Symbol (iphone.gen3) Keep this app open on your iPhone
- Symbol (visionpro) Please put on your Apple Vision Pro and launch this app there as wellCertainly! Here is a SwiftUI snippet that creates a
VStack
with labels using SF Symbols for the specified information:
import SwiftUI
struct InstructionsView: View {
var body: some View {
VStack(alignment: .leading, spacing: 20) {
Label("Keep this app open on your iPhone", systemImage: "iphone.gen3")
.font(.title2)
.padding()
Label("Please put on your Apple Vision Pro and launch this app there as well", systemImage: "visionpro")
.font(.title2)
.padding()
}
.padding()
}
}
struct InstructionsView_Previews: PreviewProvider {
static var previews: some View {
InstructionsView()
}
}
Explanation:
- Label: Each
Label
combines an SF Symbol with a text description. - systemImage: The SF Symbol identifier for the icon.
- font: The
.title2
font style to make the text larger and easily readable. - padding(): Added around each label and the overall
VStack
to ensure good spacing and layout.
This code snippet creates a simple and clean UI that presents the instructions using the specified SF Symbols and labels.Clicking Get STarted, doesn't do anythingTo ensure that clicking the "Get Started" button navigates to the PreparingView
, we need to make sure that the NavigationLink
is set up correctly within a NavigationView
. Here is the updated code:
import SwiftUI
struct WelcomeView: View {
var body: some View {
NavigationView {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
}
struct PreparingView: View {
var body: some View {
VStack {
Text("Preparing your experience...")
.font(.title)
.padding()
// Add more content for the PreparingView as needed
}
}
}
@main
struct LightsaberTrainerApp: App {
var body: some Scene {
WindowGroup {
WelcomeView()
}
}
}
Explanation of Changes:
NavigationView:
- The
NavigationView
is now wrapping the entire content of theWelcomeView
to ensure that navigation works properly.
- The
NavigationLink:
- Ensures that clicking the "Get Started" button navigates to the
PreparingView
.
- Ensures that clicking the "Get Started" button navigates to the
By wrapping the VStack
in a NavigationView
, the NavigationLink
can properly manage the navigation stack and display the PreparingView
when the button is pressed.Let's move PreparingView into it's own file. Okay, for this view, I want to re-use the image. Then I will have a horizontal view with the SF Symbol (visionpro), Symbol (ellipsis), and lastly Symbol (iphone.gen3). The visiopro should be stroked with red, the ellipsis grey and the iphone with green.
Sure! Let's move PreparingView
into its own file and update the content as per your requirements. Here are the steps and the updated code:
1. WelcomeView.swift
import SwiftUI
struct WelcomeView: View {
var body: some View {
NavigationView {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
}
struct WelcomeView_Previews: PreviewProvider {
static var previews: some View {
WelcomeView()
}
}
2. PreparingView.swift
import SwiftUI
struct PreparingView: View {
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
Image(systemName: "ellipsis")
.foregroundColor(.gray)
.font(.system(size: 40, weight: .bold))
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
}
}
3. LightsaberTrainerApp.swift
import SwiftUI
@main
struct LightsaberTrainerApp: App {
var body: some Scene {
WindowGroup {
WelcomeView()
}
}
}
Explanation:
WelcomeView.swift
:- Contains the
WelcomeView
with the navigation toPreparingView
.
- Contains the
PreparingView.swift
:- Uses the same image at the top.
- Contains a horizontal
HStack
with the SF Symbols:visionpro
,ellipsis
, andiphone.gen3
. - The symbols are styled with the specified colors and sizes.
LightsaberTrainerApp.swift
:- Initializes the app with the
WelcomeView
.
- Initializes the app with the
This structure keeps your code organized and maintains a clear separation of views. The PreparingView
file is now responsible for its content and styling, including the re-used image and the horizontally aligned SF Symbols.Is there a way to animate the ellipses with a gradient that changes over time?Yes, you can animate the ellipsis symbol with a gradient that changes over time in SwiftUI. You can use a combination of gradient and animation to achieve this effect. Here's how you can do it:
- Create a gradient that animates over time.
- Apply this gradient to the ellipsis symbol.
Here's an updated version of PreparingView.swift
that includes the animated gradient for the ellipsis symbol:
import SwiftUI
struct PreparingView: View {
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
.onAppear {
self.animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
}
}
Explanation:
State Variable:
@State private var animateGradient = false
- This state variable will be used to toggle the gradient animation.
LinearGradient:
- A
LinearGradient
is created with multiple colors. - The
startPoint
andendPoint
of the gradient are animated to create a moving effect.
- A
Animation:
- The
animation
modifier is used to apply a linear animation with a duration of 2 seconds that repeats forever and autoreverses. - The
onAppear
modifier is used to start the animation when the view appears.
- The
This setup will animate the gradient on the ellipsis symbol, giving it a dynamic and colorful effect over time.'animation' was deprecated in iOS 15.0: Use withAnimation or animation(_:value:) instead.I need to track two variables which are used across different views and are set from a different System. Connection Status is the general topic
- hasBeenConnected = false
- isConnected = false
Where would I put that and How do I connect it to PreparingView
To update the code using the recommended withAnimation
or animation(_:value:)
methods introduced in iOS 15.0, you can modify the animation logic accordingly. Here’s the updated version of the PreparingView
:
import SwiftUI
struct PreparingView: View {
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(.linear(duration: 2).repeatForever(autoreverses: true), value: animateGradient)
.onAppear {
animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
}
}
Explanation:
State Variable:
@State private var animateGradient = false
- This state variable will be used to toggle the gradient animation.
LinearGradient:
- A
LinearGradient
is created with multiple colors. - The
startPoint
andendPoint
of the gradient are animated to create a moving effect.
- A
Animation:
- The
animation(_:value:)
modifier is used to apply a linear animation with a duration of 2 seconds that repeats forever and autoreverses. - The
onAppear
modifier is used to start the animation when the view appears.
- The
This setup will animate the gradient on the ellipsis symbol, giving it a dynamic and colorful effect over time using the recommended method in iOS 15.0 and later.To manage and share state across different views in SwiftUI, you can use @StateObject
and @EnvironmentObject
. These properties allow you to maintain a single source of truth and share state between different views in a SwiftUI app. Here's how you can implement this for your connection status:
1. Create a ConnectionStatus Model
First, create a model to hold the connection status variables:
import SwiftUI
import Combine
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false
@Published var isConnected: Bool = false
}
2. Initialize and Provide the ConnectionStatus Model
In your LightsaberTrainerApp
, initialize the ConnectionStatus
model and provide it to the environment:
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
var body: some Scene {
WindowGroup {
WelcomeView()
.environmentObject(connectionStatus)
}
}
}
3. Use the ConnectionStatus in PreparingView
Now, you can use the ConnectionStatus
model in your PreparingView
and other views by accessing it through @EnvironmentObject
.
Here is the updated PreparingView
to use ConnectionStatus
:
import SwiftUI
struct PreparingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
.onAppear {
self.animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
// Display connection status
Text("Has been connected: \(connectionStatus.hasBeenConnected ? "Yes" : "No")")
.padding()
Text("Is connected: \(connectionStatus.isConnected ? "Yes" : "No")")
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
.environmentObject(ConnectionStatus())
}
}
Explanation:
ConnectionStatus Model:
- The
ConnectionStatus
class is anObservableObject
with two@Published
properties,hasBeenConnected
andisConnected
.
- The
App Initialization:
- In
LightsaberTrainerApp
, a@StateObject
instance ofConnectionStatus
is created and provided to the environment using the.environmentObject()
modifier.
- In
Using ConnectionStatus in Views:
- In
PreparingView
, the@EnvironmentObject
property wrapper is used to access the sharedConnectionStatus
instance. - The connection status values are displayed in
Text
views to demonstrate the connection status.
- In
This setup ensures that any changes to the connection status are automatically propagated to all views that use the ConnectionStatus
model.Would you call that a ViewModel?Yes, the ConnectionStatus
class can be considered a ViewModel in the context of the MVVM (Model-View-ViewModel) design pattern, which is commonly used in SwiftUI applications.
ViewModel in MVVM:
- Model: Represents the data and business logic. It can include data structures, networking code, database interactions, etc.
- View: Represents the UI and its appearance. SwiftUI views such as
WelcomeView
andPreparingView
fall into this category. - ViewModel: Acts as an intermediary between the Model and the View. It holds and manages the data required by the View, and exposes this data in a way that the View can consume and display it. The ViewModel also handles user interactions and updates the Model accordingly.
ConnectionStatus
as a ViewModel:
- Properties:
hasBeenConnected
andisConnected
represent the state of the connection. - ObservableObject: By conforming to
ObservableObject
and using@Published
properties, theConnectionStatus
ViewModel notifies the Views about changes in the state, allowing the UI to update reactively.
Full Implementation:
ConnectionStatus.swift (ViewModel)
import SwiftUI
import Combine
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false
@Published var isConnected: Bool = false
// Add any additional logic related to connection status here
}
LightsaberTrainerApp.swift (App Initialization)
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
var body: some Scene {
WindowGroup {
WelcomeView()
.environmentObject(connectionStatus)
}
}
}
WelcomeView.swift (View)
import SwiftUI
struct WelcomeView: View {
var body: some View {
NavigationView {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
}
struct WelcomeView_Previews: PreviewProvider {
static var previews: some View {
WelcomeView()
.environmentObject(ConnectionStatus())
}
}
PreparingView.swift (View)
import SwiftUI
struct PreparingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
.onAppear {
self.animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
// Display connection status
Text("Has been connected: \(connectionStatus.hasBeenConnected ? "Yes" : "No")")
.padding()
Text("Is connected: \(connectionStatus.isConnected ? "Yes" : "No")")
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
.environmentObject(ConnectionStatus())
}
}
In this setup:
- The
ConnectionStatus
class acts as a ViewModel. - It is initialized in the app entry point (
LightsaberTrainerApp
) and provided to the environment. - The views (
WelcomeView
andPreparingView
) access and react to the state managed by the ViewModel.How can I use that env variable from a class like thisclass MultipeerManager: NSObject, ObservableObject {
To use theConnectionStatus
environment object within another class such asMultipeerManager
, you need to follow these steps:
- Pass the
ConnectionStatus
instance toMultipeerManager
: You can inject theConnectionStatus
instance intoMultipeerManager
when initializing it. - Observe changes within
MultipeerManager
: Use the@Published
properties and observe changes if needed.
Here's a detailed example:
Step 1: Update MultipeerManager to Accept ConnectionStatus
First, ensure your MultipeerManager
can accept an instance of ConnectionStatus
.
import Foundation
import Combine
class MultipeerManager: NSObject, ObservableObject {
@Published var connectionStatus: ConnectionStatus
init(connectionStatus: ConnectionStatus) {
self.connectionStatus = connectionStatus
super.init()
}
// Your existing multipeer related code...
func updateConnectionStatus(hasBeenConnected: Bool, isConnected: Bool) {
connectionStatus.hasBeenConnected = hasBeenConnected
connectionStatus.isConnected = isConnected
}
}
Step 2: Initialize MultipeerManager in the App
Next, you will initialize the MultipeerManager
with the ConnectionStatus
instance in your LightsaberTrainerApp
.
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
@StateObject private var multipeerManager: MultipeerManager
init() {
let connectionStatus = ConnectionStatus()
_connectionStatus = StateObject(wrappedValue: connectionStatus)
_multipeerManager = StateObject(wrappedValue: MultipeerManager(connectionStatus: connectionStatus))
}
var body: some Scene {
WindowGroup {
WelcomeView()
.environmentObject(connectionStatus)
.environmentObject(multipeerManager)
}
}
}
Step 3: Access the MultipeerManager in Views
Now, you can access the MultipeerManager
and the ConnectionStatus
in your views using @EnvironmentObject
.
WelcomeView.swift
import SwiftUI
struct WelcomeView: View {
@EnvironmentObject var multipeerManager: MultipeerManager
var body: some View {
NavigationView {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
}
struct WelcomeView_Previews: PreviewProvider {
static var previews: some View {
WelcomeView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
PreparingView.swift
import SwiftUI
struct PreparingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@EnvironmentObject var multipeerManager: MultipeerManager
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
.onAppear {
self.animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
// Display connection status
Text("Has been connected: \(connectionStatus.hasBeenConnected ? "Yes" : "No")")
.padding()
Text("Is connected: \(connectionStatus.isConnected ? "Yes" : "No")")
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
In this setup:
- ConnectionStatus: Acts as a shared state across the app.
- MultipeerManager: Is initialized with the
ConnectionStatus
instance and can read/update the connection status. - Views: Access
ConnectionStatus
andMultipeerManager
using@EnvironmentObject
.
This allows you to manage and track the connection status within your MultipeerManager
class and across different views in a SwiftUI app.In LightsaberTrainerApp why do you call ConnectionStatus()
twice? That seems like a bugYou're correct, calling ConnectionStatus()
twice in LightsaberTrainerApp
is unnecessary and should be avoided to ensure that only a single instance of ConnectionStatus
is shared across the app. Let's fix that by ensuring we create the ConnectionStatus
instance once and use it consistently.
Here is the corrected version of LightsaberTrainerApp
:
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
@StateObject private var multipeerManager: MultipeerManager
init() {
let connectionStatus = ConnectionStatus()
_connectionStatus = StateObject(wrappedValue: connectionStatus)
_multipeerManager = StateObject(wrappedValue: MultipeerManager(connectionStatus: connectionStatus))
}
var body: some Scene {
WindowGroup {
WelcomeView()
.environmentObject(connectionStatus)
.environmentObject(multipeerManager)
}
}
}
Explanation:
Single Instance of ConnectionStatus:
- Create a single instance of
ConnectionStatus
and pass it to both the@StateObject
properties to ensure that bothconnectionStatus
andmultipeerManager
share the same instance.
- Create a single instance of
Initialize MultipeerManager with ConnectionStatus:
- Initialize
MultipeerManager
with the sharedConnectionStatus
instance in theinit()
method of theLightsaberTrainerApp
.
- Initialize
Use EnvironmentObject:
- Provide both
connectionStatus
andmultipeerManager
as environment objects to theWelcomeView
.
- Provide both
This ensures that the same ConnectionStatus
instance is used across your app, avoiding any potential bugs from having multiple instances.
Full Implementation:
ConnectionStatus.swift
import SwiftUI
import Combine
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false
@Published var isConnected: Bool = false
// Add any additional logic related to connection status here
}
LightsaberTrainerApp.swift
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
@StateObject private var multipeerManager: MultipeerManager
init() {
let connectionStatus = ConnectionStatus()
_connectionStatus = StateObject(wrappedValue: connectionStatus)
_multipeerManager = StateObject(wrappedValue: MultipeerManager(connectionStatus: connectionStatus))
}
var body: some Scene {
WindowGroup {
WelcomeView()
.environmentObject(connectionStatus)
.environmentObject(multipeerManager)
}
}
}
WelcomeView.swift
import SwiftUI
struct WelcomeView: View {
var body: some View {
NavigationView {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
Text("Lightsaber Trainer")
.font(.largeTitle)
.fontWeight(.bold)
.padding(.top, 20)
Text("Welcome to the Lightsaber Trainer app. Get ready to embark on an exciting journey to master the art of lightsaber combat.")
.font(.body)
.multilineTextAlignment(.center)
.padding()
Spacer()
NavigationLink(destination: PreparingView()) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(10)
.padding([.leading, .trailing], 40)
.padding(.bottom, 20)
}
}
}
}
}
struct WelcomeView_Previews: PreviewProvider {
static var previews: some View {
WelcomeView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
PreparingView.swift
import SwiftUI
struct PreparingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@EnvironmentObject var multipeerManager: MultipeerManager
@State private var animateGradient = false
var body: some View {
VStack {
GeometryReader { geometry in
Image("yourImageName")
.resizable()
.scaledToFill()
.frame(width: geometry.size.width, height: geometry.size.width * 0.6)
.clipped()
.edgesIgnoringSafeArea(.top)
}
.frame(height: UIScreen.main.bounds.width * 0.6)
Spacer()
HStack(spacing: 40) {
Image(systemName: "visionpro")
.foregroundColor(.red)
.font(.system(size: 40, weight: .bold))
// Ellipsis with animated gradient
Image(systemName: "ellipsis")
.font(.system(size: 40, weight: .bold))
.foregroundStyle(
LinearGradient(
gradient: Gradient(colors: [.red, .yellow, .green, .blue, .purple, .red]),
startPoint: animateGradient ? .leading : .trailing,
endPoint: animateGradient ? .trailing : .leading
)
)
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
.onAppear {
self.animateGradient.toggle()
}
Image(systemName: "iphone.gen3")
.foregroundColor(.green)
.font(.system(size: 40, weight: .bold))
}
.padding()
Spacer()
Text("Preparing your experience...")
.font(.title)
.padding()
// Display connection status
Text("Has been connected: \(connectionStatus.hasBeenConnected ? "Yes" : "No")")
.padding()
Text("Is connected: \(connectionStatus.isConnected ? "Yes" : "No")")
.padding()
}
}
}
struct PreparingView_Previews: PreviewProvider {
static var previews: some View {
PreparingView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
By following this approach, the ConnectionStatus
instance is consistently used across the entire app, ensuring that the state is shared and updated correctly.In a RoutingView I'm trying to do this. var body: some View { connectionStatus.hasBeenConnected ? LightsaberControllerView() : PreparingConnectionView() }
, but the swift compiler says Result values in '? :' expression have mismatching types 'LightsaberControllerView' and 'PreparingConnectionView'
The error you're encountering is due to the fact that the ternary conditional operator (?:
) requires both possible results to have the same type. However, LightsaberControllerView
and PreparingConnectionView
are likely two different types.
In SwiftUI, you need to ensure that the result of the conditional operator is of a single type, typically AnyView
. You can achieve this by erasing the type of the views to AnyView
. Here's how you can do it:
RoutingView.swift
import SwiftUI
struct RoutingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
var body: some View {
if connectionStatus.hasBeenConnected {
AnyView(LightsaberControllerView())
} else {
AnyView(PreparingConnectionView())
}
}
}
struct RoutingView_Previews: PreviewProvider {
static var previews: some View {
RoutingView()
.environmentObject(ConnectionStatus())
}
}
Explanation:
- AnyView: The
AnyView
type erases the specific type of the view, allowing you to return different view types from a single conditional expression. - Conditional View: The
if
statement is used to conditionally presentLightsaberControllerView
orPreparingConnectionView
, both wrapped inAnyView
.
Alternatively, you can use the Group
view to achieve the same goal without type erasure:
Alternative Approach Using Group
import SwiftUI
struct RoutingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
var body: some View {
Group {
if connectionStatus.hasBeenConnected {
LightsaberControllerView()
} else {
PreparingConnectionView()
}
}
}
}
struct RoutingView_Previews: PreviewProvider {
static var previews: some View {
RoutingView()
.environmentObject(ConnectionStatus())
}
}
Explanation:
- Group:
Group
is a container that can hold and conditionally present different views without needing to wrap them inAnyView
. - Conditional View: Inside the
Group
, theif
statement checks theconnectionStatus.hasBeenConnected
value and presents the appropriate view.
Both approaches ensure that your RoutingView
can conditionally present different views based on the connection status.For this code ```import SwiftUI
struct RoutingView: View { @EnvironmentObject var connectionStatus: ConnectionStatus @EnvironmentObject var multipeerManager: MultipeerManager
var body: some View {
VStack {
connectionStatus.hasBeenConnected ? AnyView(LightsaberControllerView()) : AnyView(PreparingConnectionView())
}
}
}
When connectionStatus.hasBeenConnected changes, I don't see this re-renderIn SwiftUI, the view should automatically re-render when an `@EnvironmentObject` changes, provided that the object conforms to the `ObservableObject` protocol and the properties are marked with `@Published`. Your current setup looks correct in terms of using `@EnvironmentObject`, but the issue might be with how the `ConnectionStatus` is updated.
Here’s a revised approach to ensure that changes in `ConnectionStatus` properly trigger a view update:
1. Ensure `ConnectionStatus` properly conforms to `ObservableObject` and uses `@Published` for properties.
2. Ensure the `RoutingView` properly observes changes in `ConnectionStatus`.
### Step 1: Ensure `ConnectionStatus` is Set Up Correctly
Ensure your `ConnectionStatus` class is properly set up as follows:
```swift
import SwiftUI
import Combine
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false
@Published var isConnected: Bool = false
}
Step 2: Ensure RoutingView
is Set Up Correctly
Ensure your RoutingView
properly observes the ConnectionStatus
changes:
import SwiftUI
struct RoutingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@EnvironmentObject var multipeerManager: MultipeerManager
var body: some View {
VStack {
if connectionStatus.hasBeenConnected {
AnyView(LightsaberControllerView())
} else {
AnyView(PreparingConnectionView())
}
}
}
}
struct RoutingView_Previews: PreviewProvider {
static var previews: some View {
RoutingView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
Step 3: Ensure LightsaberTrainerApp
is Set Up Correctly
Ensure your app is providing the ConnectionStatus
and MultipeerManager
objects correctly:
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
@StateObject private var multipeerManager: MultipeerManager
init() {
let connectionStatus = ConnectionStatus()
_connectionStatus = StateObject(wrappedValue: connectionStatus)
_multipeerManager = StateObject(wrappedValue: MultipeerManager(connectionStatus: connectionStatus))
}
var body: some Scene {
WindowGroup {
RoutingView()
.environmentObject(connectionStatus)
.environmentObject(multipeerManager)
}
}
}
Additional Debugging Tips
Ensure Property Updates: Ensure the
hasBeenConnected
property is being updated correctly in your logic. For instance, if you have a method inMultipeerManager
or another part of your code that updates this property, make sure it’s doing so correctly.Debug Output: Add print statements to verify when the property changes:
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false {
didSet {
print("hasBeenConnected changed to \(hasBeenConnected)")
}
}
@Published var isConnected: Bool = false
}
- Check View Updates: Ensure the view hierarchy is not preventing the update. Simplify the view to check for updates:
struct RoutingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
var body: some View {
VStack {
if connectionStatus.hasBeenConnected {
Text("Connected")
} else {
Text("Not Connected")
}
}
}
}
By following these steps, you should be able to ensure that RoutingView
properly re-renders when connectionStatus.hasBeenConnected
changes.Publishing changes from background threads is not allowed; make sure to publish values from the main thread (via operators like receive(on:)) on model updates.The error message indicates that you're attempting to update the @Published
properties of ConnectionStatus
from a background thread. SwiftUI requires that all UI updates happen on the main thread. To resolve this, you need to ensure that any updates to the @Published
properties occur on the main thread.
Here’s how you can modify your code to ensure that updates are performed on the main thread:
Using DispatchQueue.main.async
for Updates
Wherever you are updating connectionStatus.hasBeenConnected
or connectionStatus.isConnected
, make sure to wrap the updates in DispatchQueue.main.async
.
For example, in MultipeerManager
, if you have methods that update the ConnectionStatus
properties, wrap those updates as follows:
import Foundation
import Combine
class MultipeerManager: NSObject, ObservableObject {
@Published var connectionStatus: ConnectionStatus
init(connectionStatus: ConnectionStatus) {
self.connectionStatus = connectionStatus
super.init()
}
// Example function that updates the connection status
func updateConnectionStatus(hasBeenConnected: Bool, isConnected: Bool) {
DispatchQueue.main.async {
self.connectionStatus.hasBeenConnected = hasBeenConnected
self.connectionStatus.isConnected = isConnected
}
}
// Your existing multipeer related code...
}
Full Implementation with Main Thread Updates
ConnectionStatus.swift
import SwiftUI
import Combine
class ConnectionStatus: ObservableObject {
@Published var hasBeenConnected: Bool = false
@Published var isConnected: Bool = false
}
MultipeerManager.swift
import Foundation
import Combine
class MultipeerManager: NSObject, ObservableObject {
@Published var connectionStatus: ConnectionStatus
init(connectionStatus: ConnectionStatus) {
self.connectionStatus = connectionStatus
super.init()
}
// Example function that updates the connection status
func updateConnectionStatus(hasBeenConnected: Bool, isConnected: Bool) {
DispatchQueue.main.async {
self.connectionStatus.hasBeenConnected = hasBeenConnected
self.connectionStatus.isConnected = isConnected
}
}
// Your existing multipeer related code...
}
LightsaberTrainerApp.swift
import SwiftUI
@main
struct LightsaberTrainerApp: App {
@StateObject private var connectionStatus = ConnectionStatus()
@StateObject private var multipeerManager: MultipeerManager
init() {
let connectionStatus = ConnectionStatus()
_connectionStatus = StateObject(wrappedValue: connectionStatus)
_multipeerManager = StateObject(wrappedValue: MultipeerManager(connectionStatus: connectionStatus))
}
var body: some Scene {
WindowGroup {
RoutingView()
.environmentObject(connectionStatus)
.environmentObject(multipeerManager)
}
}
}
RoutingView.swift
import SwiftUI
struct RoutingView: View {
@EnvironmentObject var connectionStatus: ConnectionStatus
@EnvironmentObject var multipeerManager: MultipeerManager
var body: some View {
VStack {
if connectionStatus.hasBeenConnected {
AnyView(LightsaberControllerView())
} else {
AnyView(PreparingConnectionView())
}
}
}
}
struct RoutingView_Previews: PreviewProvider {
static var previews: some View {
RoutingView()
.environmentObject(ConnectionStatus())
.environmentObject(MultipeerManager(connectionStatus: ConnectionStatus()))
}
}
Explanation
- Ensure Main Thread Updates: In
MultipeerManager
, theupdateConnectionStatus
method wraps the updates toConnectionStatus
properties inDispatchQueue.main.async
to ensure they happen on the main thread. - Use of
@StateObject
and@EnvironmentObject
: TheConnectionStatus
andMultipeerManager
are correctly initialized and provided as environment objects to the views.
By following these steps, you ensure that all updates to the @Published
properties happen on the main thread, preventing the error and ensuring the UI updates correctly.
Related Chats
Lightsaber Trainer App 0.999
Preload Assets in visionOS 0.770
MultipeerManager Error Fix 0.758
Handling App Exit 0.730
Reusable SwiftUI custom view. 0.725
SwiftUI RealityKit Integration 0.708
Animate Using withAnimation 0.706
Switch to guard statement 0.703
Immersive View Phases 0.702