Node.js Log Options
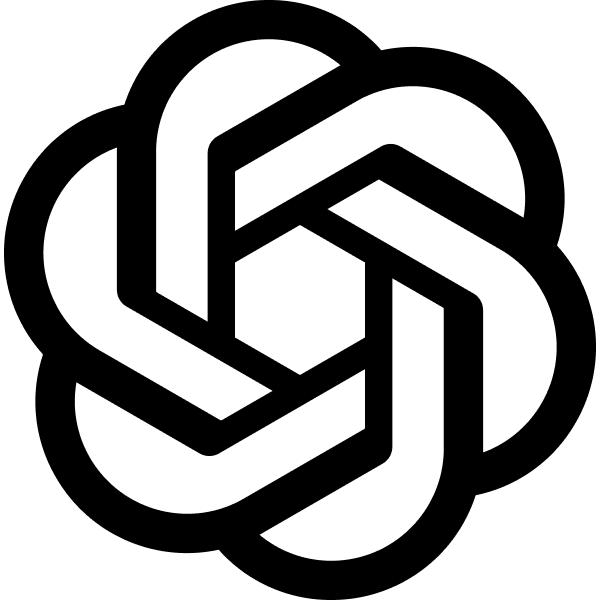
TODO: collapasable, copyable, download, etc
I want to add log level and timestamps to my ndoejs app. What is the best option there?To add log levels and timestamps to your Node.js application, you have several good options available, ranging from using built-in console methods with custom wrappers to integrating sophisticated logging libraries that offer extensive features out of the box. Here's a summary of the approaches you might consider:
1. Using Console Methods with Custom Wrappers
- Pros: Simple to implement, no external dependencies.
- Cons: Limited features, managing log levels and formats can become cumbersome for larger applications.
You can create a simple logger utility that wraps around console.log
, console.error
, etc., and adds timestamps and log levels manually. However, this approach might not be scalable for more complex needs.
2. Winston
- Pros: Highly customizable, supports multiple transports (e.g., console, file, remote services), and allows setting different log levels and formats, including timestamps.
- Cons: Slightly more overhead in learning and setup compared to basic console logging.
Winston is one of the most popular logging libraries in the Node.js ecosystem. It provides a powerful and flexible logging solution that can be easily tailored to your needs.
3. Morgan
- Pros: Specifically designed for logging HTTP requests in Node.js applications; integrates well with Express.js; easy to set up.
- Cons: Mainly focused on HTTP request logging, not a general-purpose logging tool.
Morgan is an HTTP request logger middleware for Node.js. It's very useful for logging the details of HTTP requests and responses, including timestamps. However, for logging general application logs, you might need to use it alongside another logging solution.
4. Pino
- Pros: Focuses on performance; produces logs in JSON format; supports log levels and timestamps; can be used with a variety of transports.
- Cons: JSON format might not be as human-readable as traditional text logs without additional tooling.
Pino is a very fast JSON-based logger for Node.js, ideal for production use cases where performance is critical. It also supports pretty-printing for development environments.
5. Bunyan
- Pros: Similar to Pino, offers detailed JSON logging, supports log levels, and includes a CLI tool for log viewing and filtering.
- Cons: JSON-based logging might require additional tools for log analysis and viewing.
Bunyan is another JSON logging library that provides a rich set of features for logging in Node.js applications. It’s designed for simplicity and support for structured logging.
Choosing the Best Option
- For simple projects or if you're just starting out, enhancing console logging with custom wrappers might be sufficient.
- For applications requiring detailed logs (especially with the need for log management and analysis), Winston or Pino would be a better choice.
- For logging HTTP requests in web applications, Morgan is an excellent choice.
- For high-performance applications where logging overhead needs to be minimized, Pino is recommended.
Integrating any of these libraries is generally straightforward and involves installing the library via npm, configuring it according to your needs, and then using it throughout your application to log messages at various levels.I will integrate winston. Production will run inside of a Docker container. What is the best way to configure it for the Docker log as the first way to see the output?Integrating Winston for logging in a Node.js application running inside a Docker container and optimizing it for Docker logs involves a few considerations to ensure logs are easily accessible, manageable, and observable. Docker captures the stdout and stderr streams of a container and manages them through its logging driver. To make the most of this integration, follow these best practices:
1. Log to Console
Since Docker captures stdout and stderr, configure Winston to log to the console in production. This ensures that all logs are directed to Docker's logging system, where they can be managed using Docker's log commands.
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
transports: [
new winston.transports.Console()
]
});
2. Use JSON Format
Logging in JSON format is beneficial for Dockerized applications because it facilitates log parsing and analysis by log management tools. Winston's format.json()
makes this easy.
3. Environment-Based Configuration
Differentiate log levels and formats based on the environment. In development, you might prefer pretty-printed logs for readability, but in production, JSON logs with timestamps are more practical for analysis and storage.
if (process.env.NODE_ENV !== 'production') {
logger.add(new winston.transports.Console({
format: winston.format.simple()
}));
}
4. Stream Application Logs to stdout
and stderr
Ensure that your application and any subprocess it spawns correctly stream logs to stdout
and stderr
. Avoid writing logs to files within the container unless you're using Docker volumes to manage log files outside the container's filesystem, which is generally less preferred for Dockerized applications due to additional complexity.
5. Use Docker Logging Drivers
Docker supports various logging drivers which allow you to centralize logs from all containers. By default, Docker uses the json-file
driver, which stores logs in JSON files. However, for production environments, consider using a more robust logging driver that integrates with a centralized log management system (e.g., syslog, fluentd, AWS CloudWatch, etc.).
6. Monitoring and Management
- Docker Commands: Use
docker logs [container_id]
to view logs for troubleshooting. - Centralized Logging: For production, integrate with a centralized logging solution to aggregate logs from multiple sources, making it easier to search, visualize, and analyze logs across services.
7. Health Checks and Logging
Implement health checks in your application and log health status. This is especially useful in Docker environments to quickly diagnose service availability issues.
Conclusion
By configuring Winston to log to the console in a Docker-friendly format and leveraging Docker's logging capabilities, you can create a robust logging solution that scales with your application. This setup simplifies log management and aids in the observability of your Node.js applications running in Docker containers.How would you do a winston SvelteKit integration? Show all the key files includeing one +server.js log.infoIntegrating Winston with a SvelteKit application involves setting up Winston in a way that it can be used both on the server-side part of your SvelteKit application, for example, within your endpoints or hooks, and potentially for any server-specific logic (though SvelteKit primarily runs on the client-side, it does offer server-side rendering and API routes). Below, I'll guide you through setting up Winston for server-side logging in a SvelteKit project.
Step 1: Install Winston
First, add Winston to your project dependencies:
npm install winston
Step 2: Create a Logger Configuration
Create a new file for configuring Winston. You can place this in a directory like src/lib/server
, indicating it's meant for server-side code (as SvelteKit uses Vite, which will tree-shake and not bundle this in client-side code).
// src/lib/server/logger.js
const winston = require('winston');
const logger = winston.createLogger({
level: 'info', // Default logging level
format: winston.format.combine(
winston.format.timestamp(),
winston.format.printf(({ level, message, timestamp }) => {
return `${timestamp} ${level}: ${message}`;
})
),
transports: [
new winston.transports.Console()
]
});
module.exports = logger;
Step 3: Use Logger in Your SvelteKit Endpoint
Create or modify a SvelteKit endpoint to use Winston for logging. SvelteKit endpoints are server-side and can be placed in the src/routes
directory. For example, you might have a +server.js
file for an API endpoint:
// src/routes/example/+server.js
import logger from '$lib/server/logger';
export async function GET() {
logger.info('Fetching data for /example endpoint');
// Your endpoint logic here...
return new Response(JSON.stringify({ message: 'This is an example response' }), {
headers: { 'Content-Type': 'application/json' },
});
}
Step 4: Running Your SvelteKit Application
Ensure your SvelteKit application is set up to run in an environment where Node.js is available, as the logging will occur on the server side. When you access the /example
endpoint, Winston should log the message to your console.
Notes
- Server vs. Client Logging: The above setup is for server-side logging. Client-side logging in SvelteKit would typically use console logging or a client-side logging library that sends logs to a backend service.
- Environment-Specific Configuration: Consider enhancing your Winston setup to handle different log levels or formats based on the environment (development, production, etc.). This can involve checking
process.env.NODE_ENV
and adjusting the logger configuration accordingly. - Deployment Considerations: When deploying your SvelteKit application, ensure your deployment environment supports Node.js for server-side rendering and API routes. The logging will appear in the environment where the Node.js server is running.
This setup provides a basic integration of Winston for server-side logging within a SvelteKit application, helping you monitor server-side operations, API endpoint accesses, and any server-side logic your application performs.
Node.js Log Options created at Tue Jan 20 1970 18:06:55 GMT+0000 (Coordinated Universal Time)Related Chats
Winston Logging Configuration 0.775
Logseq Plugin Development Guide 0.489
Detecting SvelteKit Environment: Logging 0.449
Luxon for Human-Friendly Durations 0.444
Debugging Docker SvelteKit Logs 0.424
Metrics Middleware for Next.js 0.393
View logs with kubectl. 0.382