Winston Logging Configuration
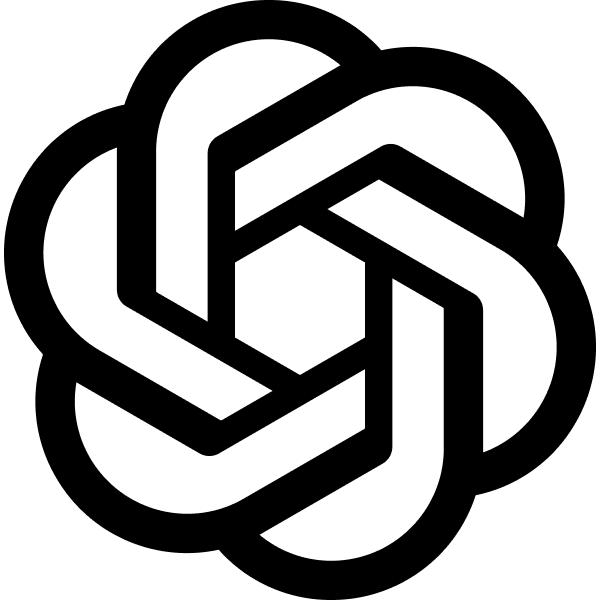
TODO: collapasable, copyable, download, etc
I want to use winston so that it has flat log lines with a timestamp, log level, etc and if I do a log like this logger.info('request input', request)
I want it to serlize that object into the log. Is taht possible? What does the winston config look like?Yes, it's possible to configure Winston, a popular logging library for Node.js, to output flat log lines with a timestamp, log level, and to serialize objects passed as log messages. Here's an overview of how you can achieve this:
Install Winston: If you haven't already, you need to install Winston in your Node.js project.
npm install winston
Configure Winston Logger: You'll need to create a logger instance and configure it to use a custom format that includes a timestamp, log level, and serializes any objects passed into the log messages.
Here's a sample configuration that demonstrates how to achieve your requirements:
const winston = require('winston');
// Define a custom format
const customFormat = winston.format.printf(({ level, message, timestamp, ...metadata }) => {
let msg = `${timestamp} [${level}] : ${message} `;
if (Object.keys(metadata).length) {
msg += JSON.stringify(metadata);
}
return msg;
});
// Create a logger instance with the custom format and settings
const logger = winston.createLogger({
level: 'info', // Minimum log level to record
format: winston.format.combine(
winston.format.timestamp(), // Add a timestamp
winston.format.errors({ stack: true }), // Ensure errors log their stack trace
winston.format.splat(), // Necessary for string interpolation
winston.format.json(), // Ensure the metadata object is properly formatted
customFormat // Use the custom format we defined earlier
),
transports: [
new winston.transports.Console(), // Log to the console
// Add other transports here as needed
],
});
// Example usage
const request = { url: 'http://example.com', method: 'GET' };
logger.info('Request input', { request });
This configuration does the following:
- Timestamp and Log Level: It includes both a timestamp and the log level in each log message.
- Serialization of Objects: If you pass an object (like the
request
object in the example) as part of the log message, it serializes this object into a JSON string and appends it to the log message. - Custom Format: The
customFormat
function constructs the log message string. It checks if there are any additional metadata properties (in case you pass extra objects/data aside from the primary log message) and serializes them into the log string.
Remember, this is a basic configuration to get you started. Winston is highly customizable, so you can adjust the transports, formats, and log levels according to your specific needs.Can winston capture the current file's name automatically?Yes, Winston can capture the current file's name automatically, but it requires a bit of custom configuration because Winston itself doesn't automatically include the file name in the log messages. You can achieve this by using Node.js's built-in __filename
variable in combination with a custom logging function or by enhancing the log metadata. However, to make this process automated and reusable across your application, you can use a custom format in Winston or wrap your logger in a function that appends the file name to each log message.
Here's a method to capture the file name automatically by creating a custom logger function that includes the file name in the log metadata:
const path = require('path');
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.errors({ stack: true }),
winston.format.json()
),
transports: [new winston.transports.Console()],
});
// Custom function to add the file name
function getLogger(module) {
const filePath = module.filename.split('/').slice(-2).join('/'); // Get the last two parts of the file path
return {
info: (message, meta) => {
logger.info(message, { ...meta, filePath });
},
error: (message, meta) => {
logger.error(message, { ...meta, filePath });
},
// Define other log levels as needed
};
}
// Usage
const customLogger = getLogger(module);
// Example log
customLogger.info('This is an info log', { additional: 'metadata' });
This example modifies the logging function to include the file path automatically by using a helper function (getLogger
) that you call in each of your modules where you want to log information. The module.filename
provides the full path to the current module's file, and you can manipulate the string to display the file path as you prefer (in this case, I've chosen to display the last two parts of the path for brevity).
This approach allows you to maintain the flexibility and power of Winston's logging capabilities while automatically including the file name in your logs for easier tracing of log statements across your application.will that log the caller's filename?The approach I previously described logs the filename where the logger is instantiated, not necessarily the caller's filename if the logger is called from a different module. This distinction is important because if you create a logger instance in one module and use it across multiple different modules, the filename logged will always be the same—the one where the logger was instantiated, not where the logger.info()
, logger.error()
, etc., methods are called.
Logging the caller's filename automatically in Node.js requires a more involved solution, as Node.js and Winston do not provide built-in support for capturing the call stack or the caller file name directly in the logging context. However, you can achieve this by capturing the call stack and parsing it to find the caller's filename. This method has performance implications and can make logging more complex, but it's a way to dynamically include the caller's filename in log messages.
Here is a conceptual example of how you might implement such functionality:
const winston = require('winston');
const path = require('path');
const { format } = winston;
// Custom format to include the caller's file name
const includeCallerInfo = format((info) => {
const stack = new Error().stack; // Capture the call stack
const stackLines = stack.split('\n');
let callerLine = stackLines[3]; // This is a bit arbitrary; you need to find the correct line
if (callerLine) {
const match = callerLine.match(/\((.*?):\d+:\d+\)$/);
if (match) {
info.caller = match[1].split(path.sep).slice(-2).join('/'); // Extract file name from path
}
}
return info;
});
const logger = winston.createLogger({
level: 'info',
format: format.combine(
includeCallerInfo(),
format.timestamp(),
format.json()
),
transports: [
new winston.transports.Console(),
],
});
// Usage example
function testLogging() {
logger.info('This message should include the caller file info');
}
testLogging();
This example uses a custom format that captures the call stack at the time of the logging call, extracts the caller's file name from it, and includes it in the log message. This method involves parsing the call stack, which can vary between Node.js versions and might require adjustments based on where you place the logger calls in your stack.
Note: This solution can significantly impact performance, especially if logging in a tight loop or in performance-critical parts of your application, due to the overhead of generating and parsing the error stack. Use this approach judiciously and test its impact on your application's performance.What is a quick bash script so that this output
src/lib/components/chat_input.svelte.debug
src/lib/components/query_builder/query_builder_inline_filter.svelte.debug
src/lib/components/graph.svelte.debug```
I could do `cp src/lib/components/chat_input.svelte.debug src/lib/components/chat_input.svelte` etc.You can achieve this with a simple bash script that reads the output of the `find` command and uses it to copy each `.debug` file to a new file without the `.debug` extension. Here's a quick script that does exactly that:
```bash
#!/bin/bash
# Find all .debug files and loop through them
find src -name '*.debug' | while read -r debugFile; do
# Construct the target file name by removing the .debug extension
targetFile="${debugFile%.debug}"
# Copy the .debug file to the target file
cp "$debugFile" "$targetFile"
echo "Copied $debugFile to $targetFile"
done
This script does the following:
- Uses
find src -name '*.debug'
to locate all.debug
files in thesrc
directory. - Pipes the output of
find
into awhile
loop that reads each line (each.debug
file path) into the variabledebugFile
. - Inside the loop,
${debugFile%.debug}
is used to remove the.debug
suffix from the file name, producing the target file name. - Uses
cp
to copy the.debug
file to the target file name (without.debug
). - Prints a message indicating what was copied to where.
You can save this script to a file, make it executable with chmod +x filename.sh
, and then run it from your terminal. Make sure to run it from the directory that contains the src
folder, or adjust the find
command to match your directory structure.
Related Chats
Node.js Log Options 0.775
Detecting SvelteKit Environment: Logging 0.541
Logseq Plugin Development Guide 0.511
Node.js POST Request Stream 0.440
Debugging Docker SvelteKit Logs 0.403
Luxon for Human-Friendly Durations 0.402
New chat 0.388