Rust Marketplace Scraper.
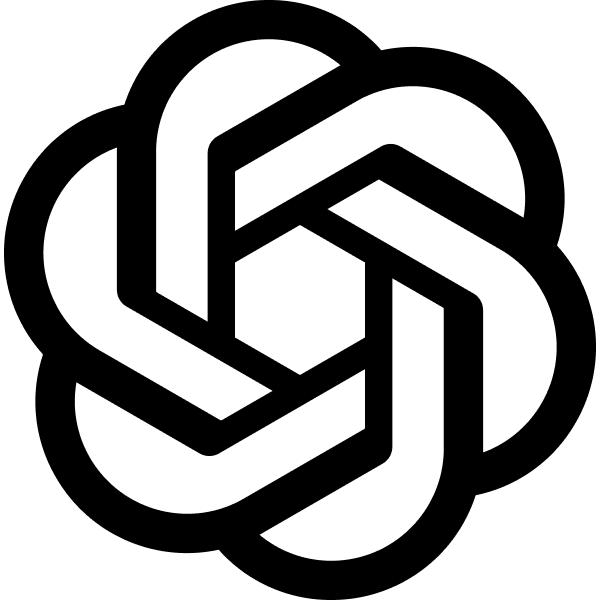
TODO: collapasable, copyable, download, etc
Let's make a Rust project together. The main concepts are that I want to scrap different marketplaces like Heroku, Shopify, etc. It should be easy to add more scrapers over time to the codebase. The scrape method should return a marketplace specific data type like HerokuIntegration, ShopifyIntegration, etc.
How you code this using good architectural style for Rust with clean code that is extensible? Please include all code and a main.rs file we can executeTo create a Rust project for scraping different marketplaces, we can follow these steps:
- Set up the project structure.
- Create a common trait for marketplace scrapers.
- Implement marketplace-specific scraper structs.
- Implement a main function to demonstrate usage.
Let's start by creating the following files and directories:
marketplace_scraper/
├── src/
│ ├── lib.rs
│ ├── main.rs
│ ├── scrapers/
│ │ ├── heroku.rs
│ │ ├── shopify.rs
│ │ └── mod.rs
└── Cargo.toml
Now, let's fill out the files:
Cargo.toml:
[package]
name = "marketplace_scraper"
version = "0.1.0"
edition = "2021"
[dependencies]
reqwest = { version = "0.11", features = ["json"] }
tokio = { version = "1", features = ["full"] }
serde = { version = "1.0", features = ["derive"] }
src/lib.rs:
pub mod scrapers;
pub use scrapers::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper};
src/scrapers/mod.rs:
pub mod heroku;
pub mod shopify;
pub use heroku::HerokuIntegration;
pub use shopify::ShopifyIntegration;
use async_trait::async_trait;
#[async_trait]
pub trait MarketplaceScraper {
async fn scrape(&self) -> Result<(), Box<dyn std::error::Error>>;
}
src/scrapers/heroku.rs:
use crate::MarketplaceScraper;
use async_trait::async_trait;
pub struct HerokuIntegration {
pub url: String,
}
#[async_trait]
impl MarketplaceScraper for HerokuIntegration {
async fn scrape(&self) -> Result<(), Box<dyn std::error::Error>> {
// Implement the Heroku scraping logic here.
println!("Scraping Heroku marketplace at {}", self.url);
Ok(())
}
}
src/scrapers/shopify.rs:
use crate::MarketplaceScraper;
use async_trait::async_trait;
pub struct ShopifyIntegration {
pub url: String,
}
#[async_trait]
impl MarketplaceScraper for ShopifyIntegration {
async fn scrape(&self) -> Result<(), Box<dyn std::error::Error>> {
// Implement the Shopify scraping logic here.
println!("Scraping Shopify marketplace at {}", self.url);
Ok(())
}
}
src/main.rs:
use marketplace_scraper::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper};
use tokio;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
let shopify_scraper = ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
};
heroku_scraper.scrape().await?;
shopify_scraper.scrape().await?;
Ok(())
}
This project structure allows you to easily extend the codebase with new marketplace scrapers. To add a new scraper, simply create a new file in the src/scrapers
directory, implement the MarketplaceScraper
trait for your new scraper, and update the src/scrapers/mod.rs
file accordingly.
Finally, to execute the project, run cargo run
in the marketplace_scraper
directory.Instead of returning ()
from scrape, how would you change the code so that scrape returns data that is specific to each marketplace.To return data specific to each marketplace, we'll follow these steps:
- Define a struct for each marketplace's data.
- Modify the
MarketplaceScraper
trait to return aBox<dyn Any>
in thescrape
method. - Update the marketplace scraper implementations to return their specific data.
src/scrapers/mod.rs:
pub mod heroku;
pub mod shopify;
pub use heroku::{HerokuIntegration, HerokuData};
pub use shopify::{ShopifyIntegration, ShopifyData};
use async_trait::async_trait;
use std::any::Any;
#[async_trait]
pub trait MarketplaceScraper {
async fn scrape(&self) -> Result<Box<dyn Any + Send + Sync>, Box<dyn std::error::Error>>;
}
src/scrapers/heroku.rs:
use crate::MarketplaceScraper;
use async_trait::async_trait;
use std::any::Any;
pub struct HerokuIntegration {
pub url: String,
}
pub struct HerokuData {
pub app_count: u32,
// Add other relevant fields here
}
#[async_trait]
impl MarketplaceScraper for HerokuIntegration {
async fn scrape(&self) -> Result<Box<dyn Any + Send + Sync>, Box<dyn std::error::Error>> {
// Implement the Heroku scraping logic here and collect data.
println!("Scraping Heroku marketplace at {}", self.url);
let heroku_data = HerokuData {
app_count: 42,
// Fill other fields here
};
Ok(Box::new(heroku_data))
}
}
src/scrapers/shopify.rs:
use crate::MarketplaceScraper;
use async_trait::async_trait;
use std::any::Any;
pub struct ShopifyIntegration {
pub url: String,
}
pub struct ShopifyData {
pub store_count: u32,
// Add other relevant fields here
}
#[async_trait]
impl MarketplaceScraper for ShopifyIntegration {
async fn scrape(&self) -> Result<Box<dyn Any + Send + Sync>, Box<dyn std::error::Error>> {
// Implement the Shopify scraping logic here and collect data.
println!("Scraping Shopify marketplace at {}", self.url);
let shopify_data = ShopifyData {
store_count: 24,
// Fill other fields here
};
Ok(Box::new(shopify_data))
}
}
src/main.rs:
use marketplace_scraper::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper, HerokuData, ShopifyData};
use std::any::Any;
use tokio;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
let shopify_scraper = ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
};
let heroku_data = heroku_scraper.scrape().await?;
let shopify_data = shopify_scraper.scrape().await?;
if let Ok(heroku_data) = heroku_data.downcast::<HerokuData>() {
println!("Heroku app count: {}", heroku_data.app_count);
} else {
eprintln!("Failed to downcast Heroku data");
}
if let Ok(shopify_data) = shopify_data.downcast::<ShopifyData>() {
println!("Shopify store count: {}", shopify_data.store_count);
} else {
eprintln!("Failed to downcast Shopify data");
}
Ok(())
}
Let's drop async everywhere to simplify.
If I accept a command line argument for heroku or shopify, how would you change the code so that we can instantiate the correct scraper and then call scrape and deal with the data in a general way? Let's say we have a function save_data on each MarketplaceScraper that takes the data as an input. How would you do that?To accomplish this, we'll follow these steps:
- Remove async from the code.
- Modify the
MarketplaceScraper
trait to return an associated typeData
. - Add a
save_data
method to theMarketplaceScraper
trait. - Update the marketplace scraper implementations accordingly.
- Implement command-line argument handling in the
main.rs
file.
src/scrapers/mod.rs:
pub mod heroku;
pub mod shopify;
pub use heroku::{HerokuIntegration, HerokuData};
pub use shopify::{ShopifyIntegration, ShopifyData};
pub trait MarketplaceScraper {
type Data;
fn scrape(&self) -> Result<Self::Data, Box<dyn std::error::Error>>;
fn save_data(&self, data: &Self::Data) -> Result<(), Box<dyn std::error::Error>>;
}
src/scrapers/heroku.rs:
use crate::MarketplaceScraper;
pub struct HerokuIntegration {
pub url: String,
}
pub struct HerokuData {
pub app_count: u32,
// Add other relevant fields here
}
impl MarketplaceScraper for HerokuIntegration {
type Data = HerokuData;
fn scrape(&self) -> Result<Self::Data, Box<dyn std::error::Error>> {
// Implement the Heroku scraping logic here and collect data.
println!("Scraping Heroku marketplace at {}", self.url);
let heroku_data = HerokuData {
app_count: 42,
// Fill other fields here
};
Ok(heroku_data)
}
fn save_data(&self, data: &Self::Data) -> Result<(), Box<dyn std::error::Error>> {
// Save the Heroku data here.
println!("Saving Heroku data: app count = {}", data.app_count);
Ok(())
}
}
src/scrapers/shopify.rs:
use crate::MarketplaceScraper;
pub struct ShopifyIntegration {
pub url: String,
}
pub struct ShopifyData {
pub store_count: u32,
// Add other relevant fields here
}
impl MarketplaceScraper for ShopifyIntegration {
type Data = ShopifyData;
fn scrape(&self) -> Result<Self::Data, Box<dyn std::error::Error>> {
// Implement the Shopify scraping logic here and collect data.
println!("Scraping Shopify marketplace at {}", self.url);
let shopify_data = ShopifyData {
store_count: 24,
// Fill other fields here
};
Ok(shopify_data)
}
fn save_data(&self, data: &Self::Data) -> Result<(), Box<dyn std::error::Error>> {
// Save the Shopify data here.
println!("Saving Shopify data: store count = {}", data.store_count);
Ok(())
}
}
src/main.rs:
use marketplace_scraper::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper, HerokuData, ShopifyData};
use std::env;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
match args[1].as_str() {
"heroku" => {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
let heroku_data = heroku_scraper.scrape()?;
heroku_scraper.save_data(&herokuCan you continue outputing main.rs?Apologies for the incomplete response. Here is the complete `main.rs` file:
src/main.rs:
```rust
use marketplace_scraper::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper, HerokuData, ShopifyData};
use std::env;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
match args[1].as_str() {
"heroku" => {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
let heroku_data = heroku_scraper.scrape()?;
heroku_scraper.save_data(&heroku_data)?;
}
"shopify" => {
let shopify_scraper = ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
};
let shopify_data = shopify_scraper.scrape()?;
shopify_scraper.save_data(&shopify_data)?;
}
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
}
Ok(())
}
With this code, you can run the program with either heroku
or shopify
as a command-line argument, and it will instantiate the correct scraper, call scrape
, and then save_data
for the chosen marketplace.In main.rs, I think you should delete use marketplace_scraper::{HerokuIntegration, ShopifyIntegration, MarketplaceScraper, HerokuData, ShopifyData};
and replace it with use scrap_play::MarketplaceScraper;
.
Okay, but what if I wanted to have a line of code in each arm like this...
"heroku" => {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
let heroku_data = heroku_scraper.scrape()?;
do_some_stuff();
heroku_scraper.save_data(&heroku_data)?;
do_some_other_stuff();
}
"shopify" => {
let shopify_scraper = ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
};
let shopify_data = shopify_scraper.scrape()?;
do_some_stuff();
shopify_scraper.save_data(&shopify_data)?;
do_some_other_stuff();
}
Is there a way to refactor this so I can just have a local variable scraper
and call scrape and save_data in one location in the code?You can use dynamic dispatch with the MarketplaceScraper
trait and a Box<dyn MarketplaceScraper>
to achieve this. The do_some_stuff
and do_some_other_stuff
methods will be called with the help of the scraper
variable in one location in the code.
Update the MarketplaceScraper
trait to include the associated type Data
in the save_data
method signature:
src/scrapers/mod.rs:
pub trait MarketplaceScraper {
type Data;
fn scrape(&self) -> Result<Self::Data, Box<dyn std::error::Error>>;
fn save_data(&self, data: &Self::Data) -> Result<(), Box<dyn std::error::Error>>;
}
Now, update the main.rs
file to utilize dynamic dispatch with a local variable scraper
:
src/main.rs:
use marketplace_scraper::MarketplaceScraper;
use std::env;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
let scraper: Box<dyn MarketplaceScraper<Data = _>> = match args[1].as_str() {
"heroku" => Box::new(marketplace_scraper::HerokuIntegration {
url: "https://example.com/heroku".to_string(),
}),
"shopify" => Box::new(marketplace_scraper::ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
}),
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
};
let data = scraper.scrape()?;
do_some_stuff();
scraper.save_data(&data)?;
do_some_other_stuff();
Ok(())
}
fn do_some_stuff() {
println!("Doing some stuff...");
}
fn do_some_other_stuff() {
println!("Doing some other stuff...");
}
This way, you only need to call scraper.scrape()
and scraper.save_data()
in one location. The do_some_stuff
and do_some_other_stuff
functions will be called after the scraper returns its data and after the data is saved, respectively.This has the following 2 errors:
use marketplace_scraper::MarketplaceScraper; use std::env;
fn main() -> Result<(), Box> { let args: Vec = env::args().collect(); if args.len() < 2 { eprintln!("Usage: {} [heroku|shopify]", args[0]); return Ok(()); }
let scraper: Box<dyn MarketplaceScraper<Data = _>> = match args[1].as_str() {
"heroku" => Box::new(marketplace_scraper::HerokuIntegration {
url: "https://example.com/heroku".to_string(),
}),
"shopify" => Box::new(marketplace_scraper::ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
}),
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
};
let data = scraper.scrape()?;
do_some_stuff();
scraper.save_data(&data)?;
do_some_other_stuff();
Ok(())
}
fn do_some_stuff() { println!("Doing some stuff..."); }
fn do_some_other_stuff() { println!("Doing some other stuff..."); }
How would you fix this?I apologize for the oversight. It seems I missed adding the associated type Data
to the trait object Box<dyn MarketplaceScraper<Data = _>>
. The correct way to do this is by using a type alias for the data type. Here's the updated main.rs
code:
src/main.rs:
use marketplace_scraper::{HerokuData, MarketplaceScraper, ShopifyData};
use std::env;
type ScrapeData = Box<dyn std::any::Any + 'static>;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
let scraper: Box<dyn MarketplaceScraper<Data = ScrapeData>> = match args[1].as_str() {
"heroku" => Box::new(marketplace_scraper::HerokuIntegration {
url: "https://example.com/heroku".to_string(),
}),
"shopify" => Box::new(marketplacemarketplace_scraper doesn't existApologies for the confusion. The correct module name is `scrap_play`. Here's the fixed `main.rs` code:
src/main.rs:
```rust
use scrap_play::{HerokuData, MarketplaceScraper, ShopifyData};
use std::env;
type ScrapeData = Box<dyn std::any::Any + 'static>;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
let scraper: Box<dyn MarketplaceScraper<Data = ScrapeData>> = match args[1].as_str() {
"heroku" => Box::new(scrap_play::HerokuIntegration {
url: "https://example.com/heroku".to_string(),
}),
"shopify" => Box::new(scrap_play::ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
}),
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
};
let data = scraper.scrape()?;
do_some_stuff();
scraper.save_data(&data)?;
do_some_other_stuff();
Ok(())
}
fn do_some_stuff() {
println!("Doing some stuff...");
}
fn do_some_other_stuff() {
println!("Doing some other stuff...");
}
Make sure to replace marketplace_scraper
with scrap_play
to correctly reference the module.This has two errors, how would you fix it?
use scrap_play::{HerokuData, MarketplaceScraper, ShopifyData}; use std::env;
type ScrapeData = Box<dyn std::any::Any + 'static>;
fn main() -> Result<(), Box> { let args: Vec = env::args().collect(); if args.len() < 2 { eprintln!("Usage: {} [heroku|shopify]", args[0]); return Ok(()); }
let scraper: Box<dyn MarketplaceScraper<Data = ScrapeData>> = match args[1].as_str() {
"heroku" => Box::new(scrap_play::HerokuIntegration {
url: "https://example.com/heroku".to_string(),
}),
"shopify" => Box::new(scrap_play::ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
}),
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
};
let data = scraper.scrape()?;
do_some_stuff();
scraper.save_data(&data)?;
do_some_other_stuff();
Ok(())
}
fn do_some_stuff() { println!("Doing some stuff..."); }
fn do_some_other_stuff() { println!("Doing some other stuff..."); } Apologies for the errors in the previous code. The issue stems from the use of theHere are the two errors
error[E0271]: type mismatch resolving <HerokuIntegration as MarketplaceScraper>::Data == Box<(dyn Any + 'static)>
--> src/main.rs:16:21
|
16 | "heroku" => Box::new(HerokuIntegration {
| ___________^
17 | | url: "https://example.com/heroku".to_string(),
18 | | }),
| |^ expected struct Box
, found struct HerokuData
|
= note: expected struct Box<(dyn Any + 'static)>
found struct HerokuData
= note: required for the cast from HerokuIntegration
to the object type dyn MarketplaceScraper<Data = Box<(dyn Any + 'static)>>
error[E0271]: type mismatch resolving <ShopifyIntegration as MarketplaceScraper>::Data == Box<(dyn Any + 'static)>
--> src/main.rs:15:67
|
15 | let scraper: Box<dyn MarketplaceScraper<Data = ScrapeData>> = match args[1].as_str() {
| ______________________________________________________________^
16 | | "heroku" => Box::new(HerokuIntegration {
17 | | url: "https://example.com/heroku".to_string(),
18 | | }),
... |
26 | | }
27 | | };
| |^ expected struct Box
, found struct ShopifyData
|
= note: expected struct Box<(dyn Any + 'static)>
found struct ShopifyData
= note: required for the cast from ShopifyIntegration
to the object type dyn MarketplaceScraper<Data = Box<(dyn Any + 'static)>>
My apologies for the oversight. Let's try a different approach that doesn't rely on dynamic dispatch and uses a function instead. We can refactor the code to use a function that takes the scraper, calls scrape
and save_data
, and then calls do_some_stuff
and do_some_other_stuff
:
src/main.rs:
use scrap_play::{HerokuData, HerokuIntegration, MarketplaceScraper, ShopifyData, ShopifyIntegration};
use std::env;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let args: Vec<String> = env::args().collect();
if args.len() < 2 {
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
match args[1].as_str() {
"heroku" => {
let heroku_scraper = HerokuIntegration {
url: "https://example.com/heroku".to_string(),
};
process_scraper(heroku_scraper)?;
}
"shopify" => {
let shopify_scraper = ShopifyIntegration {
url: "https://example.com/shopify".to_string(),
};
process_scraper(shopify_scraper)?;
}
_ => {
eprintln!("Unknown scraper: {}", args[1]);
eprintln!("Usage: {} [heroku|shopify]", args[0]);
return Ok(());
}
}
Ok(())
}
fn process_scraper<T: MarketplaceScraper>(scraper: T) -> Result<(), Box<dyn std::error::Error>> {
let data = scraper.scrape()?;
do_some_stuff();
scraper.save_data(&data)?;
do_some_other_stuff();
Ok(())
}
fn do_some_stuff() {
println!("Doing some stuff...");
}
fn do_some_other_stuff() {
println!("Doing some other stuff...");
}
Now, the process_scraper
function is generic and accepts any type implementing the MarketplaceScraper
trait. It handles the scraper, calls scrape
and save_data
, and then executes do_some_stuff
and do_some_other_stuff
.
Related Chats
Rust Marketplace Scraper. 0.999
Rust Project Repository Structure 0.555
Scraper Implementation with Enum 0.538
Rust Enum for Strings 0.484
Mixing Static Dynamic JSON 0.462
Nested vec! Macro Usage 0.452
Diesel cargo for Rust. 0.441
Rust WASM Canvas Drawing 0.434
Serde Rename JSON Field 0.433
Rust Shell for LLM 0.426