Mixing Static Dynamic JSON
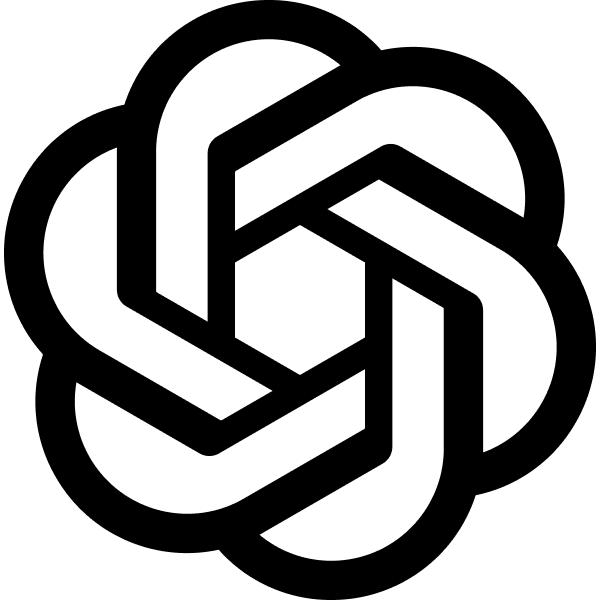
TODO: collapasable, copyable, download, etc
In Rust when using serde_json, can I have a mixture of statically typed and dynamically typed objects?Yes, in Rust, you can have a mixture of statically typed and dynamically typed objects when using the serde_json
crate. You can use the serde_json::Value
type to represent a dynamically typed JSON value, while using structs to represent statically typed data.
Here's an example demonstrating the use of both statically typed and dynamically typed objects with serde_json:
use serde_json::{Result, Value};
use serde::{Deserialize, Serialize};
// Statically typed struct
#[derive(Serialize, Deserialize, Debug)]
struct Person {
name: String,
age: u32,
}
fn main() -> Result<()> {
let static_data = r#"
{
"name": "Alice",
"age": 30
}
"#;
let dynamic_data = r#"
{
"hobbies": ["reading", "swimming"],
"address": {
"city": "New York",
"state": "NY"
}
}
"#;
// Deserialize static data into a Person struct
let person: Person = serde_json::from_str(static_data)?;
// Deserialize dynamic data into a serde_json::Value
let value: Value = serde_json::from_str(dynamic_data)?;
println!("Statically typed data: {:?}", person);
println!("Dynamically typed data: {:?}", value);
Ok(())
}
In this example, we have a statically typed Person
struct representing a person's name and age. We also have a dynamically typed JSON object containing the person's hobbies and address, represented by a serde_json::Value
.
We deserialize the static data into a Person
struct, while the dynamic data is deserialized into a serde_json::Value
. This way, we can work with both statically typed and dynamically typed JSON objects using serde_json.I mean, what if the Person struct had a member metadata: Value
, would that work? So that JSON like this could be consumed:
{ "name": "Alice", "age": 30, "metadata": { "hobbies" ... }
Yes, you can have a serde_json::Value
as a member of a struct to represent a part of the JSON data with a dynamic type. Here's an example:
use serde_json::{Result, Value};
use serde::{Deserialize, Serialize};
// Statically typed struct with a dynamically typed field (metadata)
#[derive(Serialize, Deserialize, Debug)]
struct Person {
name: String,
age: u32,
metadata: Value,
}
fn main() -> Result<()> {
let data = r#"
{
"name": "Alice",
"age": 30,
"metadata": {
"hobbies": ["reading", "swimming"],
"address": {
"city": "New York",
"state": "NY"
}
}
}
"#;
// Deserialize the data into a Person struct
let person: Person = serde_json::from_str(data)?;
println!("Person: {:?}", person);
Ok(())
}
In this example, the Person
struct has a metadata
field of type serde_json::Value
. This allows you to have a mixture of statically typed and dynamically typed JSON data within a single struct. The metadata
field will store the dynamic JSON data, allowing you to deserialize and work with JSON objects that have a combination of static and dynamic types.
Related Chats
Mixing Static Dynamic JSON 1.000
Serde Rename JSON Field 0.655
Rust Marketplace Scraper. 0.462
Rust Enum for Strings 0.456
New chat 0.412
Scraper Implementation with Enum 0.398
Type Chats: ChatSession Creation 0.388
Nested vec! Macro Usage 0.386
MD5 Hash in Rust 0.384