Serde Rename JSON Field
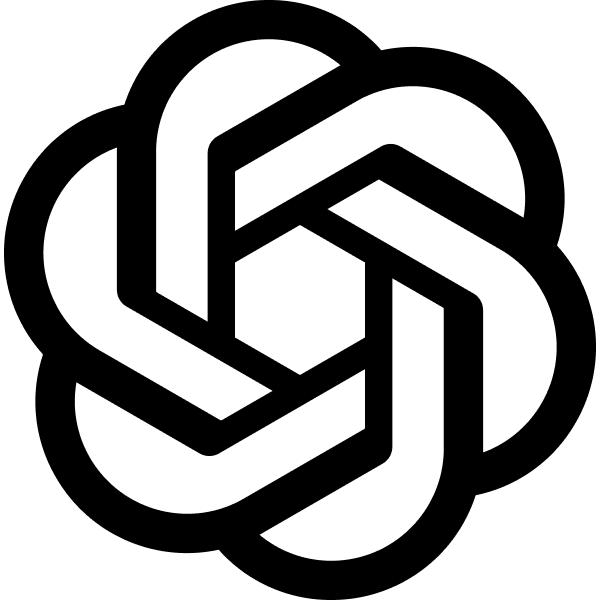
TODO: collapasable, copyable, download, etc
In Rust using serde_json how can I tell serde that pub struct PageJsonBlob { pub inital_options: InitialOptions, }
that intial_options should look like INITIAL_OPTIONS in the JSON?To tell Serde to deserialize the field initial_options
from the JSON key INITIAL_OPTIONS
, you can use the #[serde(rename = "INITIAL_OPTIONS")]
attribute on the field.
Here's the updated PageJsonBlob
struct:
use serde::{Deserialize, Serialize};
#[derive(Serialize, Deserialize, Debug)]
pub struct PageJsonBlob {
#[serde(rename = "INITIAL_OPTIONS")]
pub initial_options: InitialOptions,
}
// Your InitialOptions struct implementation
#[derive(Serialize, Deserialize, Debug)]
pub struct InitialOptions {
// Fields of InitialOptions
}
The #[serde(rename = "INITIAL_OPTIONS")]
attribute tells Serde to use the specified string ("INITIAL_OPTIONS" in this case) as the JSON key when serializing and deserializing the initial_options
field.Using Clap, how can I give an argument a short name d
and the long name crawl_day
?In Clap, you can give an argument both a short and a long name by using the short
and long
methods when defining the argument. Here's an example of how to do this:
use clap::{App, Arg};
fn main() {
let matches = App::new("My App")
.arg(
Arg::new("crawl_day")
.short('d')
.long("crawl_day")
.value_name("CRAWL_DAY")
.about("Specify the crawl day")
.takes_value(true),
)
.get_matches();
// You can access the value of the argument like this:
if let Some(crawl_day) = matches.value_of("crawl_day") {
println!("How would I do that with `Cli::parse()` instead?To use `clap` with a struct-based approach and `Cli::parse()`, you'll need to use the `clap_derive` crate to derive the `Clap` trait for your struct. Here's an example:
1. Add `clap_derive` to your `Cargo.toml`:
```toml
[dependencies]
clap = "3.0"
clap_derive = "3.0"
- Define the struct with the appropriate attributes for the short and long names:
use clap::Clap;
#[derive(Clap, Debug)]
#[clap(name = "My App", version = "1.0", author = "Your Name")]
struct Cli {
/// Specify the crawl day
#[clap(short = 'd', long = "crawl_day")]
crawl_day: String,
}
fn main() {
let cli = Cli::parse();
// Access the crawl_day field like this:
println!("Crawl day: {}", cli.crawl_day);
}
In this example, the #[clap(short = 'd', long = "crawl_day")]
attribute sets the short and long names for the crawl_day
field. When you run your application, you can use either -d
or --crawl_day
to provide a value for this argument.
Related Chats
Serde Rename JSON Field 1.000
Mixing Static Dynamic JSON 0.655
Scraper Implementation with Enum 0.506
Rust Enum for Strings 0.505
New chat 0.459
Rust Marketplace Scraper. 0.433
Fix Return Local Reference 0.380
MD5 Hash in Rust 0.379
Fix HttpResponse Type Mismatch 0.364
Nested vec! Macro Usage 0.359