Force Sync Read Atom.
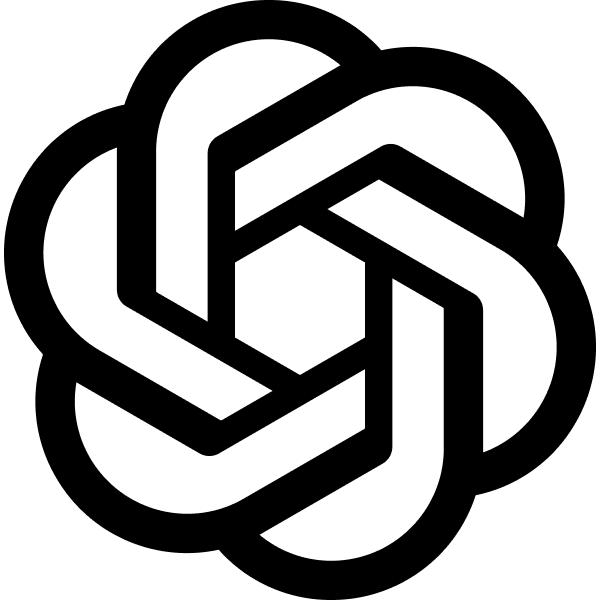
TODO: collapasable, copyable, download, etc
In jotai, can I force the fresh reading of an atom state, instead of letting React trigger the codepath again later?In Jotai, a state management library for React, atoms represent pieces of state. The primary way to interact with these atoms is through hooks, like useAtom
, which subscribe to atom state changes and trigger re-renders in your component when the state updates.
To address your specific question about forcing a fresh reading of an atom state:
Synchronous Updates: Jotai inherently works synchronously when updating and reading atom states within React components. When you update an atom using the
set
function fromuseAtom
, the component will re-render with the updated state unless specific optimizations (like React'smemo
oruseMemo
) prevent it. This means that as soon as the state updates, the latest state is available for reading.Forcing Reactivity: If you need to ensure that a component or a piece of logic always reads the latest state immediately after it has been set, you might consider restructuring your component to reactively respond to state changes, which is the default behavior in React. Make sure that no optimizations are blocking the re-renders.
Using Effects: Sometimes, if you need to perform actions synchronously after state changes, you can use
useEffect
to listen to changes in the atom state and act upon them. This ensures that your code runs immediately after React has committed the updates to the screen.const [value, setValue] = useAtom(myAtom); useEffect(() => { // This code will run after `value` has been updated and the component has re-rendered console.log('Atom value updated to: ', value); }, [value]); // Dependency on the atom's value
Directly Reading Atom State Outside React: If you need to read the atom state outside of a React component (not common and typically not recommended in usual front-end patterns), you can use Jotai's core functions directly in conjunction with a store. However, this is advanced usage and should be handled with care as it can lead to unmanaged side effects and harder-to-follow code.
If you provide more specific details about what you're trying to achieve, I can offer more targeted advice or code examples!
Force Sync Read Atom. created at Tue Jan 20 1970 20:09:00 GMT+0000 (Coordinated Universal Time)Related Chats
Provider vs useAtom: when? 0.744
Jotai with React Query 0.668
Atom Access Methods 0.652
Memo Once, No Dependencies 0.607
No need setFoo. 0.553
Use Effect vs Component Body 0.545
Reset Saving State Strategically 0.538
Svelte: Force New Component 0.443
Wrap in useCallback Hook 0.421