Dismiss Pop-up on Click
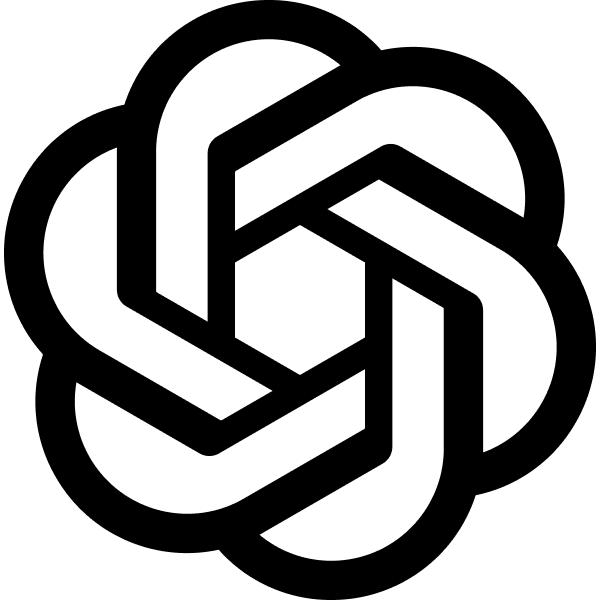
TODO: collapasable, copyable, download, etc
In my React application, I have a pop-up that should be dismissed if the user clicks anywhere else in the app. What is the best way to accomplish that?To achieve the functionality where a pop-up in your React application is dismissed when the user clicks anywhere else in the app, you can implement an event listener that checks if the click occurred outside of the pop-up component. Here’s a step-by-step guide on how to do this:
1. Define the Pop-up Component
First, create a pop-up component that can be controlled via a prop to show or hide it.
import React, { useRef, useEffect } from 'react';
function Popup({ isOpen, onClose, children }) {
const popupRef = useRef();
useEffect(() => {
function handleClickOutside(event) {
if (popupRef.current && !popupRef.current.contains(event.target)) {
onClose(); // call the onClose function if the click is outside the popup
}
}
if (isOpen) {
document.addEventListener('mousedown', handleClickOutside);
}
return () => {
document.removeEventListener('mousedown', handleClickOutside);
};
}, [isOpen, onClose]);
if (!isOpen) return null;
return (
<div ref={popupRef}>
{children}
</div>
);
}
2. Use the Pop-up Component
Integrate the pop-up into your main component. You can manage the state of the pop-up (open or closed) through a state variable.
import React, { useState } from 'react';
import Popup from './Popup';
function App() {
const [isPopupOpen, setPopupOpen] = useState(false);
const togglePopup = () => {
setPopupOpen(!isPopupOpen);
};
return (
<div>
<button onClick={togglePopup}>Toggle Pop-up</button>
<Popup isOpen={isPopupOpen} onClose={() => setPopupOpen(false)}>
<h2>Pop-up Content</h2>
<p>More content here...</p>
</Popup>
</div>
);
}
Explanation
- useRef: This hook is used to reference the pop-up DOM element. It allows us to check if a click is inside or outside this element.
- useEffect: The effect hook manages the lifecycle of the event listener:
- Add the event listener when the pop-up is open.
- Remove the event listener when the component unmounts or when the pop-up closes to clean up and prevent memory leaks.
- handleClickOutside: This function checks if the click is outside the pop-up. It uses the
contains
method to determine if the clicked element is outside theref
of the pop-up.
This method ensures that the pop-up will close when the user clicks anywhere outside of it, enhancing the user interface experience by behaving as users typically expect for modals and pop-ups.If I have a click that lands on another part of the app, how would I know to toggle the popup?To handle the scenario where a click anywhere else in the app should toggle the popup (either opening or closing it depending on the context), you can use a more global approach in handling click events. Essentially, you'll want to set up a way to detect clicks throughout your application and determine whether those clicks are inside or outside the popup component. This can be achieved by attaching a global event listener and managing the state accordingly.
Here's how you can implement this:
Step 1: Setup Global Event Listener
Modify the main app component to listen for all mousedown
or click
events at the top level. This global event handler will decide if the popup should be toggled.
import React, { useState, useEffect, useRef } from 'react';
import Popup from './Popup';
function App() {
const [isPopupOpen, setPopupOpen] = useState(false);
const appRef = useRef(null);
useEffect(() => {
function handleOutsideClick(event) {
// Check if the click is inside the app and toggle the popup based on the click location
if (appRef.current && !appRef.current.contains(event.target)) {
if (isPopupOpen) {
setPopupOpen(false); // Close popup if it's open and click is outside
}
} else {
// Here, manage specific logic if you want to open the popup when clicking on specific elements
// For example, a button inside appRef that toggles the popup:
// if (event.target.id === 'popupToggleButton') {
// setPopupOpen(!isPopupOpen);
// }
}
}
// Attach the event listener to the document to capture all clicks
document.addEventListener('mousedown', handleOutsideClick);
return () => {
document.removeEventListener('mousedown', handleOutsideClick);
};
}, [isPopupOpen]);
return (
<div ref={appRef}>
<button id="popupToggleButton" onClick={() => setPopupOpen(!isPopupOpen)}>
Toggle Pop-up
</button>
<Popup isOpen={isPopupOpen} onClose={() => setPopupOpen(false)}>
<h2>Pop-up Content</h2>
<p>More content here...</p>
</Popup>
</div>
);
}
Explanation:
- Global Event Listener: The event listener is set on the entire document to catch all clicks. It checks if the click is outside the application's main container (
appRef
). If it is and the popup is open, it closes the popup. - Refinement: The conditional inside the
handleOutsideClick
function can be refined based on your application's specific requirements. For example, you might only want to toggle the popup when certain buttons are clicked or certain parts of the application are interacted with. - Popup Component: No changes needed in the popup component itself; it remains responsible for rendering its content and handling its local behavior.
This approach ensures that your application has full control over when and how the popup is displayed based on global interactions, which is flexible and maintains a good separation of concerns between global behaviors and component-specific behaviors.
Dismiss Pop-up on Click created at Tue Jan 20 1970 20:16:12 GMT+0000 (Coordinated Universal Time)Related Chats
Dismiss Pop-up on Click 1.000
Use Effect vs Component Body 0.557
Listen for Command+K. 0.526
Wrap in useCallback Hook 0.513
React JSX Conditional Rendering 0.478
Reset Saving State Strategically 0.414
React TypeScript Component: Props & Render 0.412
No need setFoo. 0.408