CLI Program Readiness Detection
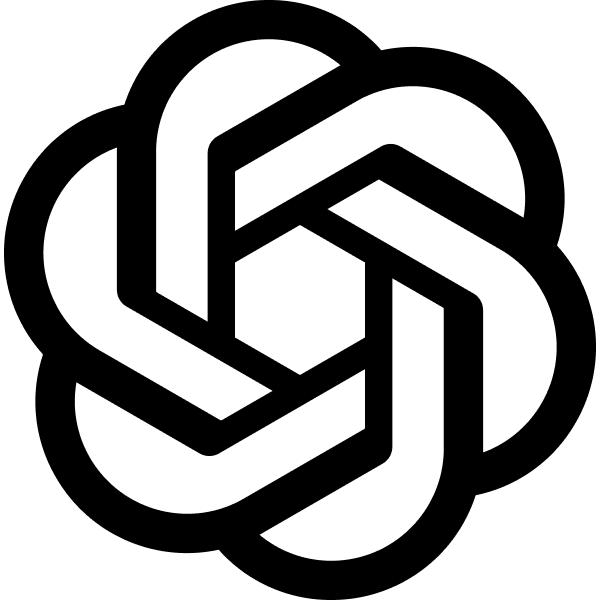
TODO: collapasable, copyable, download, etc
I have a CLI program that can take input. It takes a while to startup. What is the best strategy for detecting when it is ready for input?When dealing with a Command Line Interface (CLI) program that takes a while to start up and you need to detect when it's ready to receive input, there are several strategies you can consider:
Output Monitoring: If the program outputs a specific message or prompt when it's ready, you can monitor its standard output (stdout) for this message. This can be done by reading the program's output in a separate thread or process and looking for the specific text that indicates readiness.
Timeouts and Polling: Implement a timeout mechanism where you periodically send a test command or signal to the CLI program to check if it's ready. If the program responds appropriately, you can assume it's ready. Be careful with this method to avoid flooding the program with too many requests.
Log File Monitoring: If the program writes logs to a file, you can monitor this log file for specific entries that indicate readiness. This approach is similar to output monitoring but uses file I/O instead of direct stdout reading.
Socket or IPC Mechanism: If the program supports inter-process communication (IPC) or socket communication, you can use these mechanisms to query its status. This is more complex but offers a robust solution, especially for programs that are designed with IPC capabilities.
Fixed Wait Time: As a simpler approach, you could wait for a fixed amount of time that is known to be sufficient for the program to start. This is not the most reliable method, as the startup time might vary, but it can be a practical solution in some cases.
Startup Scripts or Wrappers: Create a script or wrapper around the CLI program that can detect its readiness and signal it to your main application. This could involve combining some of the above methods and providing a simplified interface to your main application.
Check for Process Stability: Sometimes, simply checking if the process has stopped consuming CPU resources or has reached a stable state in terms of memory usage can be an indicator that it has finished initialization.
Each of these strategies has its own pros and cons, and the best approach depends on the specific characteristics of the CLI program you're working with. For example, if the program reliably outputs a specific message when ready, output monitoring would be the most straightforward approach. However, if the program's startup behavior is less predictable, a combination of methods might be necessary.For output monitoring, does it have to be in a seperate thread?For output monitoring, using a separate thread is a common and effective approach, but it's not the only way. The necessity of using a separate thread depends on the architecture of your program and how you handle I/O operations. Here are some considerations:
Single-threaded (Blocking) Approach: If you have a simple script or application where you can afford to block the main thread while waiting for the CLI program to be ready, you can do the output monitoring in the main thread. This is simpler but less flexible, as it halts other operations in your program while waiting for the CLI output.
Multi-threaded (Non-blocking) Approach: In more complex applications, especially those with a GUI or those that need to perform other tasks concurrently, using a separate thread for output monitoring is beneficial. It allows your main thread to remain responsive and perform other tasks while another thread handles the output monitoring.
Asynchronous I/O: Modern programming languages often support asynchronous I/O operations, which can be used to monitor output without blocking the main thread and without explicitly creating a new thread. This approach can be more scalable and efficient, especially for I/O-bound tasks.
External Tools or Libraries: Some programming environments or external libraries offer tools to handle this kind of task. For example, you could use a library that abstracts the complexity of asynchronous or threaded I/O, making it easier to implement output monitoring without deep knowledge of threading or asynchronous programming.
Event-driven Models: In an event-driven model, your program could be set up to trigger a function or method whenever new output is detected from the CLI program. This can be a clean and efficient way to handle output, especially in frameworks or languages that support event-driven programming well.
In summary, whether to use a separate thread for output monitoring depends on the complexity of your application, the need for concurrency, and your comfort with multi-threading or asynchronous programming. For simple scripts, a blocking single-threaded approach might be sufficient, but for more complex applications, a non-blocking approach using threads, asynchronous I/O, or event-driven models is usually more appropriate.Please output a basic Rust program that executes /Users/ozten/Downloads/mistral-7b-instruct-v0.1-Q4_K_M-main.llamafile -i
. Do not use threads. This is single threaded. Wait until you see this line - If you want to submit another line, end your input with '\'.
At that point, the CLI is ready for input. Send it "What is 2 + 2?"
Then print out the output that it sends abck. Lastly, create a REPL where we will send it whatevever the user types into the CLI.To create a basic Rust program that executes the given CLI program, waits for a specific line of output, sends a command, and then acts as a REPL, we can use Rust's standard library, particularly the std::process::{Command, Stdio}
and std::io::{self, Write, BufRead}
modules.
The following Rust program demonstrates this approach:
use std::process::{Command, Stdio};
use std::io::{self, Write, BufRead};
use std::error::Error;
fn main() -> Result<(), Box<dyn Error>> {
let mut child = Command::new("/Users/ozten/Downloads/mistral-7b-instruct-v0.1-Q4_K_M-main.llamafile")
.arg("-i")
.stdin(Stdio::piped())
.stdout(Stdio::piped())
.spawn()?;
let child_stdin = child.stdin.as_mut().ok_or("Failed to open stdin")?;
let mut child_stdout = io::BufReader::new(child.stdout.ok_or("Failed to open stdout")?);
let mut line = String::new();
while child_stdout.read_line(&mut line)? > 0 {
if line.contains("If you want to submit another line, end your input with '\\'") {
break;
}
line.clear();
}
writeln!(child_stdin, "What is 2 + 2?")?;
// Read the response
line.clear();
child_stdout.read_line(&mut line)?;
println!("Output: {}", line);
// REPL - Read-Eval-Print Loop
let stdin = io::stdin();
loop {
print!("Enter command: ");
io::stdout().flush()?;
let mut input = String::new();
stdin.read_line(&mut input)?;
writeln!(child_stdin, "{}", input.trim_end())?;
// Read and print the response from the CLI program
line.clear();
child_stdout.read_line(&mut line)?;
println!("Output: {}", line);
}
}
This program does the following:
- It starts the CLI program with arguments and sets up pipes for stdin and stdout.
- It reads lines from the CLI program's stdout until it finds the specified line.
- It sends the command "What is 2 + 2?" to the CLI program.
- It reads and prints the output from the CLI program.
- It enters a REPL loop, allowing the user to type commands which are sent to the CLI program, and then prints the responses.
Please note that this is a basic implementation. Error handling and other aspects like handling the program's stderr or dealing with unexpected outputs from the CLI program should be considered for a more robust solution. Additionally, the program assumes that each command/response is a single line, which might not be the case for all CLI programs.In Rust, how can I have a string buffer and on each output add it to the buffer. When I am done, then covnert the buffer back to a string?In Rust, you can use a String
as a buffer to accumulate output, and since a String
is already a string, there's no need to convert it back at the end. The String
type in Rust is mutable and can be appended to, making it suitable for use as a buffer.
Here's a basic example of how you can do this:
Initialize the String Buffer: You start by creating an empty
String
. This will be your buffer.Append to the Buffer: As you receive output, you append it to the buffer using the
push_str
method (if you are appending string slices) orpush
method (if you are appending characters).Use the Buffer: At the end, your buffer is already a
String
, so you can use it directly without any conversion.
Here's a simple code snippet illustrating this:
fn main() {
let mut buffer = String::new(); // Step 1: Initialize the buffer
// Step 2: Append to the buffer
buffer.push_str("Hello, ");
buffer.push_str("world!");
buffer.push('\n');
buffer.push_str("This is a test buffer.");
// Step 3: Use the buffer
// The buffer is already a String, so you can use it directly
println!("Buffer content:\n{}", buffer);
}
In this example, buffer
is a String
that starts empty. We then append various strings and characters to it. Finally, we print the contents of the buffer. This is a very common pattern in Rust for building up strings incrementally.
Remember that each push_str
or push
call potentially involves memory allocation. If you know in advance an approximate size of the final string, you can use String::with_capacity(capacity)
to pre-allocate enough memory and avoid repeated allocations.
Related Chats
Rust Shell for LLM 0.343
Tests untuk initializeNewThread 0.337
Debugging Docker SvelteKit Logs 0.332
Bash Command: Hello World 0.326
Mock `console.error` test. 0.316
Check Closed Controller State 0.302
Rust Text File Parser 0.288