New chat
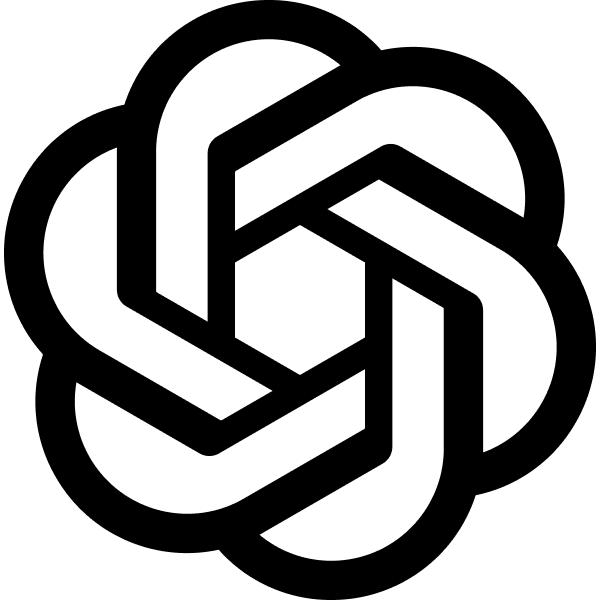
TODO: collapasable, copyable, download, etc
I'm writing a SwiftUI mac app. I will run some code that will output a string. I will then display that string. How do I replace "Hello, world!" with a variable that updates after the code finishes running? ```// // ContentView.swift // Zero2NeuralNetworkHero // // Created by Austin King on 2/22/24. //
import SwiftUI
struct ContentView: View { var body: some View { VStack { Image(systemName: "globe") .imageScale(.large) .foregroundStyle(.tint) Text("Hello, world!") } .padding() } }
#Preview {
ContentView()
}
```I want to create a new Swift file BigramModel and git it a run function. How would I incoporate that?To incorporate a BigramModel
with a run
function into your SwiftUI macOS app, you'll need to follow these steps:
Create the
BigramModel
Swift File:- Create a new Swift file in your project and name it
BigramModel.swift
. - Define a class or struct named
BigramModel
in this file. - Add a
run
function toBigramModel
that performs your desired operation and returns a string.
- Create a new Swift file in your project and name it
Update
ContentView
to UseBigramModel
:- Modify
ContentView
to hold an instance ofBigramModel
and to call itsrun
function. - Use SwiftUI's state management to update the UI when the
run
function completes.
- Modify
Below is an example implementation of these steps:
Step 1: Creating BigramModel.swift
// BigramModel.swift
import Foundation
class BigramModel {
func run() -> String {
// Your code logic here
return "Output from BigramModel"
}
}
Step 2: Updating ContentView.swift
You'll need to introduce state management to ContentView
to react to changes. Here’s how you can do it by using @State
for simple state management or @ObservableObject
for more complex scenarios. I'll show you the @ObservableObject
method, which is more suited for model updates.
First, modify BigramModel
to conform to ObservableObject
and update your run
method to be compatible with asynchronous execution:
// BigramModel.swift
import Foundation
class BigramModel: ObservableObject {
@Published var output: String = "Hello, world!"
func run() {
// Simulate doing some work
DispatchQueue.main.asyncAfter(deadline: .now() + 2) { // Simulate async work
self.output = "Output from BigramModel"
}
}
}
Then, update ContentView.swift
to use BigramModel
:
// ContentView.swift
import SwiftUI
struct ContentView: View {
@StateObject private var bigramModel = BigramModel()
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundStyle(.tint)
Text(bigramModel.output) // Use the `output` property
}
.padding()
.onAppear {
bigramModel.run() // Call run when the view appears
}
}
}
// Preview
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
This setup creates a BigramModel
instance as an observable object. The ContentView
subscribes to updates to bigramModel.output
. When bigramModel.run()
is called (e.g., when the view appears), it eventually updates output
, and the ContentView
automatically reflects this change.
This approach allows you to separate your model logic (BigramModel
) from your view (ContentView
), adhering to SwiftUI's data flow principles.How would I make a big text area that uses line breaks for formatting in SwiftUI?In SwiftUI, to create a large text area that supports line breaks and multiline text input, you can use the TextEditor
view. TextEditor
provides a way to edit multiline, scrollable plain text. It's useful for text input fields where users need to enter a significant amount of text, such as comments, notes, or any form of longer text communication.
Here’s how you can incorporate a TextEditor
into your SwiftUI view to create a big text area:
import SwiftUI
struct ContentView: View {
@State private var text: String = "Type something here..."
var body: some View {
VStack {
Text("Your Text:")
.font(.headline)
TextEditor(text: $text)
.foregroundColor(.primary) // Text color
.padding() // Padding around the text editor
.border(Color.gray, width: 1) // Border around the text editor
.frame(height: 300) // Adjust the height as needed
}
.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Explanation:
@State private var text: String
: This state variable holds the text entered in theTextEditor
. The initial value is set to guide the user.TextEditor(text: $text)
: TheTextEditor
is initialized with a binding to thetext
state variable. This means theTextEditor
will display the current value oftext
, and any changes made within theTextEditor
will update thetext
variable.- Styling: I added some basic styling like foreground color, padding, a border, and a fixed height to make the text area visually distinct and large enough for substantial input.
- Frame Height: Adjust the
frame(height:)
modifier to change the size of the text area according to your needs.
TextEditor
will automatically wrap text to the next line when the user reaches the end of the visible area, adhering to the natural line breaks. Users can also manually insert line breaks by pressing Return or Enter (depending on the keyboard).
This setup provides a straightforward way to include a large, multiline text input area in your SwiftUI application, suitable for both macOS and iOS targets.How can I achive this output? Hello WOrld
To achieve the output where "Hello" and "World" are on separate lines, you can simply use a string with a line break (\n
) between the words. In SwiftUI, if you're using a Text
view to display this string, it will respect the line break and render the text accordingly.
Here's how you can modify a SwiftUI view to display "Hello" and "World" on separate lines:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello\nWorld") // Using a line break
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In this example, the Text
view is initialized with a string that contains a line break (\n
) between "Hello" and "World". SwiftUI's Text
will render the text with "Hello" on the first line and "World" on the second line, exactly as you specified.Why do I need the $
?The $
symbol in SwiftUI is used for two-way data binding. It allows you to create a two-way connection between a UI component and its underlying data model. When you use $
with a property, it means that:
The UI can display the current value of the property: Your UI component (e.g.,
Text
,TextField
,TextEditor
) will show the current value of the property it is bound to. This is the "read" part of the binding.The UI can update the property's value based on user input: When the user interacts with the UI component (e.g., types into a
TextField
orTextEditor
), the new value will be stored back into the property. This is the "write" part of the binding.
Without $
, you are passing a value to a property, which means the UI will only display the value but won't be able to update it. With $
, you are passing a binding to the property, enabling both display and update capabilities.
Example:
Consider a TextField
in SwiftUI:
@State private var name: String = ""
TextField("Enter your name", text: $name)
Here, name
is a state variable that holds a string. By using $name
in the TextField
, you are creating a two-way binding:
- The
TextField
will display the current value ofname
. - If the user types into the
TextField
,name
will be updated with the new text.
This mechanism is fundamental to SwiftUI's declarative approach, where the UI is a function of the state. Changes in state automatically reflect in the UI, and changes in the UI (due to user interaction) automatically update the state.What if I only want to read?If you only want to read a value and display it in your SwiftUI view without the need for two-way data binding, you simply use the variable without the $
symbol. This approach is used when you want to present data to the user, but the user is not meant to modify it directly through the UI component in question.
For example, if you have a String
property and you want to display its value in a Text
view, you would do it like this:
@State private var message: String = "Hello, World!"
var body: some View {
Text(message) // No $ symbol, as this is read-only
}
In this case, message
is displayed in a Text
view. Since Text
views are inherently read-only (the user cannot edit them directly), there's no need for a two-way binding, and thus, no need for the $
symbol.
This read-only approach is applicable to any situation where the data is being displayed for informational purposes, such as labels, titles, or any static text content. The SwiftUI framework updates the UI to reflect changes in these properties automatically, without requiring user interaction to trigger updates.I have names.txt
which I would like to read and distribute with my app. How do I do that?"drop it under your app's target " what is the apps target?In the context of developing an app, particularly when using Xcode for iOS or macOS app development, the "app's target" refers to the specific build configuration used to compile the app for a device or simulator. A target includes settings and instructions for compiling the app's code, including information like the app's name, its deployment version, and which files are included in the build.
When instructions suggest you "drop it under your app's target," it means you should add the file (names.txt
in your case) to your project in a way that it becomes part of the app's bundle. This is so the file is included with your app when it's built and distributed, allowing your app to access and read the file at runtime.
Here's how to add names.txt
to your app's target in Xcode:
Open Your Project in Xcode:
- Launch Xcode and open the project for your app.
Add the File to Your Project:
- Drag
names.txt
from Finder into the Xcode project navigator. You can also use the menu option:File
>Add Files to "YourProjectName"...
.
- Drag
Ensure the File is Added to Your Target:
- When you add the file, a dialog will appear asking how you want to add the file. Ensure that the "Copy items if needed" checkbox is checked so the file is copied into your project directory (if it's not already there).
- Important: Make sure the checkbox next to your app's target under "Add to targets" is checked. This step is what it means to "drop it under your app's target." Checking this box includes the file in your app's build so it's available when your app runs.
Access the File in Your Code:
- Once added, you can access
names.txt
from your Swift code using the Bundle API. For example:if let filePath = Bundle.main.path(forResource: "names", ofType: "txt") { do { let contents = try String(contentsOfFile: filePath) print(contents) } catch { // Handle the error print("Error reading the file") } }
- Once added, you can access
Following these steps ensures that names.txt
is included in your app's bundle, making it accessible at runtime. The file will be part of your app when it's distributed, whether through the App Store, TestFlight, or any other means.In Swift, how do I initialize an array to be 27 arrays with each having an array of 27 zeroes? (27x27 matrix)In Swift, you can initialize a 27x27 matrix (an array of arrays) where each inner array contains 27 zeroes using the Array initialization syntax. This can be done concisely with the following code:
let matrix = Array(repeating: Array(repeating: 0, count: 27), count: 27)
Here's what's happening in this code:
Array(repeating: 0, count: 27)
: This creates an array of 27 zeroes. It uses theArray
initializer that takes a repeating value (0
in this case) and acount
of how many times to repeat it (27 times, for the 27 columns).Array(repeating: ..., count: 27)
: This wraps the first part in anotherArray
initializer, effectively creating an array of 27 elements, where each element is the array of 27 zeroes from the first step. This results in a 27x27 matrix of zeroes.
This approach is useful for initializing matrices for algorithms, grid layouts, or any situation where you need a multi-dimensional array with a fixed size and initial value.I have this function func append(msg: String.SubSequence) { self.output += String(msg) + "\n" }
, but I would rather not supply msg:
when I call it. Is that possible in Swift? Yes, in Swift, you can define a function with parameters that don't require argument labels when you call the function. This is achieved by using an underscore _
before the parameter name in the function definition. This tells Swift that no argument label is needed for that parameter when the function is called.
Here's how you can modify your append
function to not require the msg:
label when calling it:
func append(_ msg: String.SubSequence) {
self.output += String(msg) + "\n"
}
With this definition, you can call append
without specifying the msg:
label, like so:
append("Your message here")
This makes the function call more concise and is particularly useful when the context of the argument is clear without the need for a label.In swift, how can I iterate line 2 characters at a time?To iterate over a string two characters at a time in Swift, you can use various approaches depending on your exact needs and preferences. One common approach is to use a combination of indices and subscripting to extract pairs of characters as you iterate through the string. Here's a basic example:
let string = "Hello, World!"
// Ensure there are at least 2 characters to iterate over
guard string.count >= 2 else { fatalError("String needs to be at least 2 characters long") }
// Iterate over the string indices, stepping by 2 each time
for index in stride(from: string.startIndex, to: string.endIndex, by: 2) {
let nextIndex = string.index(index, offsetBy: 1, limitedBy: string.endIndex) ?? string.endIndex
let pair = String(string[index..<nextIndex])
print(pair)
}
In this example:
stride(from:to:by:)
is used to move through the string's indices two steps at a time.string.index(index, offsetBy: 1, limitedBy: string.endIndex)
is used to safely get the index that is one position away from the current one, ensuring it does not go past the end of the string. If it does reach the end,string.endIndex
is used to prevent an out-of-bounds error.String(string[index..<nextIndex])
creates a substring of each character pair, which is then printed.
This approach ensures that if your string has an odd number of characters, the last iteration will include the final character by itself, preventing any out-of-bounds errors.
If your use case specifically requires handling each character pair in a particular way (e.g., transforming them, storing them in an array), you can modify the body of the loop to suit your needs.Same, but I will deal with each characetr, so let ch1
, etcIf you need to deal with each character individually within the pairs as you iterate through the string two characters at a time, you can modify the approach to explicitly extract each character in the pair. Here’s how you can do it:
let string = "Hello, World!"
// Ensure there are at least 2 characters to iterate over
guard string.count >= 2 else { fatalError("String needs to be at least 2 characters long") }
// Iterate over the string indices, stepping by 2 each time
for index in stride(from: string.startIndex, to: string.endIndex, by: 2) {
let nextIndex = string.index(index, offsetBy: 1, limitedBy: string.endIndex) ?? string.endIndex
// Safely extract the first character of the pair
let ch1 = string[index]
// Attempt to extract the second character if it exists
var ch2: Character?
if nextIndex != string.endIndex {
ch2 = string[nextIndex]
}
// Now you can deal with ch1 and ch2 as individual characters
print("ch1: \(ch1)", terminator: "")
if let ch2 = ch2 {
print(", ch2: \(ch2)")
} else {
print("") // Just print ch1 if ch2 does not exist
}
}
In this example:
ch1
is always assigned the character at the current index, because we know it exists due to the guard statement ensuring the string has at least two characters.ch2
is conditionally assigned the next character ifnextIndex
is not equal tostring.endIndex
. This is important for handling cases where the string has an odd number of characters, ensuring the code doesn't attempt to access beyond the end of the string.- The characters
ch1
andch2
(ifch2
exists) are then available for individual processing as needed within the loop.
This approach allows you to work with each character in the pairs individually, providing flexibility for a wide range of processing tasks.eferencing instance method 'next()' on 'StrideToIterator' requires that 'Substring.Index' (aka 'String.Index') conform to 'Strideable'The error you're encountering suggests that there's a misunderstanding in the use of StrideToIterator
with String.Index
. In Swift, String.Index
does not conform to Strideable
, which means you cannot directly use stride(from:to:by:)
with string indices as I inaccurately suggested. I apologize for the confusion. Swift's handling of string indices is more nuanced due to the complexity of Unicode characters and their representation in memory.
To iterate through a string two characters at a time, handling each character individually, you can use a different approach that manually increments indices. Here's a corrected method:
let string = "Hello, World!"
var currentIndex = string.startIndex
while currentIndex < string.endIndex {
let ch1 = string[currentIndex]
var ch2: Character?
// Move to the next index for the second character
let nextIndex = string.index(after: currentIndex)
if nextIndex < string.endIndex {
ch2 = string[nextIndex]
currentIndex = string.index(after: nextIndex) // Prepare for the next pair
} else {
currentIndex = string.endIndex // End the loop if there's no second character
}
// Now you can deal with ch1 and ch2 as individual characters
print("ch1: \(ch1)", terminator: "")
if let ch2 = ch2 {
print(", ch2: \(ch2)")
} else {
print("") // Just print ch1 if ch2 does not exist
}
}
This method manually increments currentIndex
through the string. It safely checks if a second character exists for each pair and adjusts currentIndex
accordingly to either point to the next pair or to the end of the string if it reaches the last character.
ch1
is the first character of the current pair.ch2
is conditionally set to the second character if it exists.- The loop then prints
ch1
andch2
(ifch2
exists), showing how you can individually deal with each character.
This approach avoids the issue with Strideable
and properly handles string iteration in Swift, accommodating strings with an odd number of characters as well.How can I run this project with xcodebuild
directly instead of using XCode?To run a project using xcodebuild
from the command line instead of using the Xcode IDE, you'll need to use Terminal (or another command-line interface). The xcodebuild
command allows you to build and test your Xcode projects and workspaces from the command line. It's a powerful tool for automation and for environments where you might not use the Xcode IDE directly, such as continuous integration (CI) systems.
Here's a general guide on how to use xcodebuild
to build and run your project:
1. Open Terminal
Navigate to your project's directory using the cd
command.
2. List Your Targets, Build Configurations, and Schemes
Before you can build your project, you'll need to know the names of the build schemes and configurations. If you're unsure, you can list all available schemes and configurations in your project or workspace by running:
xcodebuild -list
3. Build Your Project
To build your project, use the following command, replacing YourSchemeName
with the name of your scheme:
xcodebuild -scheme YourSchemeName build
If you are working with a workspace instead of a project, you'll also need to specify the workspace:
xcodebuild -workspace YourWorkspaceName.xcworkspace -scheme YourSchemeName build
4. Run Your Project
xcodebuild
does not directly support launching your application. However, you can build it with xcodebuild
and then run the compiled app using a different command or manually. For macOS apps, you can find the executable in your build products folder (typically under build/Debug
or build/Release
), and you can run it directly from there.
For iOS, simulating an app from the command line can be achieved with xcrun simctl
. Here's a basic example of how you might launch an app on a simulator:
First, ensure your simulator is running. You can list available simulators with:
xcrun simctl list devices
Then, install the app on the simulator using its bundle ID:
xcrun simctl install booted your.app.bundle.id
Finally, launch your app:
xcrun simctl launch booted your.app.bundle.id
Note: Replace your.app.bundle.id
with your app's actual bundle identifier.
Additional Notes
For more complex projects, you might need to specify additional parameters such as the configuration (Debug, Release) or the SDK (e.g.,
iphonesimulator
,iphoneos
).Continuous integration environments and automated scripts often use
xcodebuild
for building and testing apps.xcodebuild
has many options and parameters. For more detailed information, consult thexcodebuild
man page by typingman xcodebuild
in the Terminal or refer to the Xcode Build System Guide in the Apple Developer documentation.
Related Chats
New chat 1.000
SwiftUI View with Model 0.575
Add Function to SwiftUI. 0.532
StateObject Error Resolution 0.477
Looping in Swift 0.470
Pass Data to SwiftUI Window. 0.464
Thread with Timer: Swift 0.459
Troubleshoot `hasBeenConnected` SwiftUI 0.456
SwiftUI iOS App: Features 0.447
Pass Data to SwiftUI 0.446