Pass Data to SwiftUI Window.
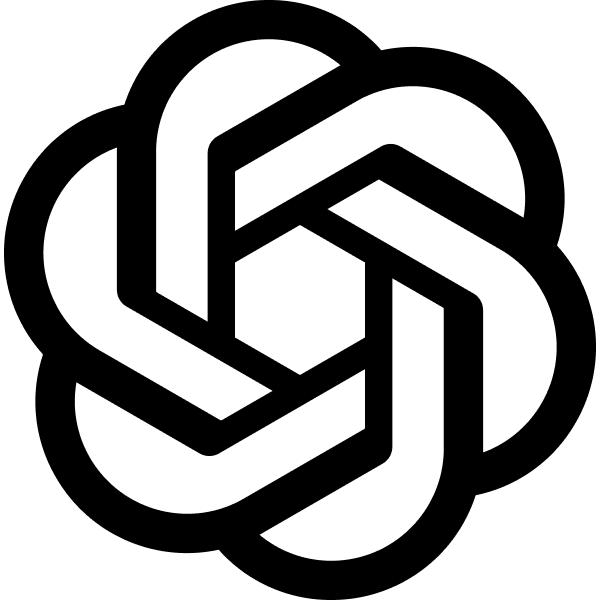
TODO: collapasable, copyable, download, etc
I'm writing a SwiftUI app. I call openWindow(id: 'frameMaker') And that Window is configured with ```WindowGroup(id: "frame") {
Frame()
}```. How can I pass an argument to Frame so that I can pass a selected image and display it in that view code?In SwiftUI, to pass an argument to a view when you're working within a WindowGroup
context, you typically initialize your view with the necessary parameters directly within the view's initializer. However, in the context of opening a new window programmatically and needing to pass data to it, you'd need to handle the data flow slightly differently, as SwiftUI doesn't provide a direct method to pass arguments to views in a new window through the openWindow
call.
One approach to achieve your goal is to use a shared view model that both the calling context and the new window's view can access. This shared view model can hold the information needed by the new window (e.g., the selected image) and be observed by the view within the new window for changes. Here's how you could structure this:
Define a Shared ViewModel: Create a view model that holds the selected image or any other data you wish to pass to the new window. This view model should be observable, typically by conforming to the
ObservableObject
protocol.Instantiate and Share the ViewModel: Ensure that the instance of this view model is accessible both from the place where you're calling
openWindow(id:)
and within theFrame
view that you're looking to pass the data to.Observe the ViewModel in Your View: Make your
Frame
view observe the shared view model, so it can react to changes in the data you're passing (e.g., the selected image).
Here is an example implementation of these steps:
Step 1: Define a Shared ViewModel
import SwiftUI
import Combine
class SharedViewModel: ObservableObject {
@Published var selectedImage: UIImage?
}
Step 2: Instantiate and Share the ViewModel
Create an instance of SharedViewModel
in a location that's accessible both by your window opening logic and by the Frame
view. If your app structure allows, you might hold this in your app's environment or in a higher-level view model.
Step 3: Observe the ViewModel in Your View
Modify your Frame
view to accept and observe the SharedViewModel
.
struct Frame: View {
@ObservedObject var viewModel: SharedViewModel
var body: some View {
Image(uiImage: viewModel.selectedImage ?? UIImage())
.resizable()
// Configure your image view here
}
}
Integrating with the WindowGroup
When configuring your WindowGroup
, make sure to pass the shared view model to your Frame
view. Given SwiftUI's declarative nature, you'd typically do this by directly initializing Frame
with the view model when you're setting up your window groups. However, since you're opening windows programmatically with openWindow(id:)
, ensure that the Frame
view or its parent view is configured to use the shared view model you've set up.
WindowGroup(id: "frame") {
Frame(viewModel: sharedViewModel)
}
Keep in mind, the exact implementation will depend on your app's architecture, particularly how you manage and pass around your shared view model. This pattern allows you to maintain a clean separation of concerns while still dynamically passing data across different parts of your SwiftUI application.I have two git users for GitHub on this laptop. I forget how to switch between them. I have ~/.ssh/id_ed25519_ozten and the .pub file. How can I switch to using that identity? How can I validate in git or with GitHub from the CLI that I'm using the correct identity?Switching between different SSH identities for GitHub involves a few steps to ensure that your Git operations use the correct SSH key for authentication. You can configure SSH to use a specific key for GitHub and verify the setup by testing the SSH connection. Here's how to do it:
1. Configure SSH to Use the Specific Key
Create or edit the ~/.ssh/config
file to specify which SSH key should be used for GitHub. If you don't already have a config
file in your ~/.ssh
directory, you can create one.
# Open the SSH config file in your favorite text editor, e.g., nano, vim, etc.
nano ~/.ssh/config
Add the following configuration, adjusting as necessary for your specific setup and key file:
# GitHub configuration
Host github.com-ozten
HostName github.com
User git
IdentityFile ~/.ssh/id_ed25519_ozten
IdentitiesOnly yes
This configuration tells SSH to use the specific key (id_ed25519_ozten
) when connecting to GitHub under the alias github.com-ozten
. The IdentitiesOnly yes
option ensures that only the specified identity file is used for authentication, ignoring any other identities you might have loaded into your SSH agent.
2. Update Git Repository Configuration
For the Git repository where you want to use this specific identity, you'll need to change the repository's remote URL to use the alias defined in your SSH config (github.com-ozten
).
Navigate to your Git repository and use the following command to change the remote URL, replacing your_username/your_repository
with your actual GitHub username and repository name:
git remote set-url origin [email protected]:your_username/your_repository.git
This tells Git to use the SSH configuration for github.com-ozten
when interacting with your GitHub repository.
3. Validate SSH Configuration
To test that your SSH setup is correct and that you can authenticate with GitHub using your specified identity, use the following SSH command:
ssh -T [email protected]
If everything is set up correctly, you should receive a welcome message from GitHub indicating that you've successfully authenticated. Note that the username shown in this message is not important; it's the successful authentication that matters.
4. Validate with GitHub from the CLI
To further validate that you're using the correct identity with GitHub, you can try performing a Git operation that requires authentication, such as pushing a commit or fetching from a private repository. If the operation succeeds without authentication errors, your setup is correct.
Remember, you can use this approach to configure and switch between multiple SSH keys for GitHub by adding more configurations to your ~/.ssh/config
file and updating your repository remotes accordingly.What about git author for the current repoTo configure the Git author information (name and email) for the current repository, which is different from the SSH identity used for authentication, you can use the git config
command within your repository's directory. This allows you to specify the name and email address that will be associated with your commits in that specific repository.
Here's how you can set the author name and email for the current repository:
Open your terminal and navigate to your repository where you want to set the author information.
Set the author name for the current repository by running:
git config user.name "Your Name"
Replace
"Your Name"
with the name you wish to appear as the author of your commits.Set the author email for the current repository by running:
git config user.email "[email protected]"
Replace
"[email protected]"
with the email address you wish to be associated with your commits.
These commands will add entries to the .git/config
file of your repository, setting the user.name
and user.email
values only for that repository. This means your global Git configuration (~/.gitconfig
) will not be affected, and these authorship settings will only apply to commits made in the current repository.
Validate Git Author Configuration
To validate or view the configured Git author information for the current repository, you can use the git config
command to read the configured values:
For the author name, run:
git config user.name
For the author email, run:
git config user.email
These commands will display the name and email address configured for the current repository. If you've set these correctly, the output should match the name and email address you intended to use for your commits.
Note on Commits
Remember, changing the author information in your Git configuration affects only future commits. If you need to change the author information on previous commits, you would need to use more advanced Git operations, such as git rebase
for individual commits or a script for multiple commits, which should be done with caution to avoid disrupting your repository history, especially if the commits have already been pushed to a shared repository.
Related Chats
Pass Data to SwiftUI Window. 1.000
Pass Data to SwiftUI 0.928
SwiftUI View with Model 0.599
Frame Photos with SwiftUI 0.560
Control Window Size: VisionOS 0.505
StateObject Error Resolution 0.503
Add Function to SwiftUI. 0.494
SwiftUI App, Centered Image 0.483
Reusable SwiftUI custom view. 0.472
New chat 0.465