New chat
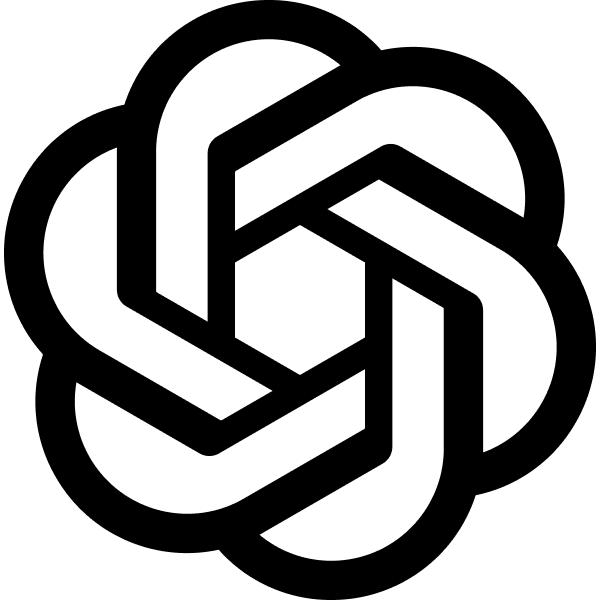
TODO: collapasable, copyable, download, etc
Can you generate Rust code which calls the Notion SDK library to create a blank page by page name?Certainly! The Rust code below uses the Notion SDK to create a blank page with a given page name:
First, you'll want to add the Notion SDK to your Cargo.toml
:
[dependencies]
notion = "X.Y.Z" # Replace X.Y.Z with the appropriate version.
Then, you can use the following Rust code:
extern crate notion;
use notion::Client;
use notion::models::Page;
use notion::models::PropertyValue;
fn create_blank_page(client: &Client, parent_page_id: &str, page_name: &str) -> Result<(), Box<dyn std::error::Error>> {
let mut new_page = Page::new();
new_page.parent_type = Some("database_id".into());
new_page.parent_id = Some(parent_page_id.into());
let title_property_value = PropertyValue::Title(vec![page_name.into()]);
new_page.properties.insert("Name".into(), title_property_value); // Assuming the property name is "Name"
client.pages().create(&new_page)?;
Ok(())
}
fn main() {
let client = Client::new("<YOUR_NOTION_SECRET_API_KEY>");
// Replace "YOUR_DATABASE_ID" with the database ID where you want to create the page and "My New Page" with the desired page name.
match create_blank_page(&client, "YOUR_DATABASE_ID", "My New Page") {
Ok(_) => println!("Page created successfully!"),
Err(e) => eprintln!("Failed to create the page: {:?}", e),
}
}
Replace <YOUR_NOTION_SECRET_API_KEY>
with your Notion Secret API key and "YOUR_DATABASE_ID"
with the database ID where you want to create the page.
Note that the exact details and structure of the Notion SDK library may vary, so make sure to consult the official Notion SDK documentation for Rust (if available) for any updates or changes.
New chat created at Tue Jan 20 1970 15:43:30 GMT+0000 (Coordinated Universal Time)Related Chats
Serde Rename JSON Field 0.459
Rust Marketplace Scraper. 0.405
Rust WASM Canvas Drawing 0.382
Mixing Static Dynamic JSON 0.371
Nested vec! Macro Usage 0.340
Diesel cargo for Rust. 0.312
Fix HttpResponse Type Mismatch 0.310
Convert Entity to ModelEntity 0.298
Rust Enum for Strings 0.295