Store Subscription Error Fix
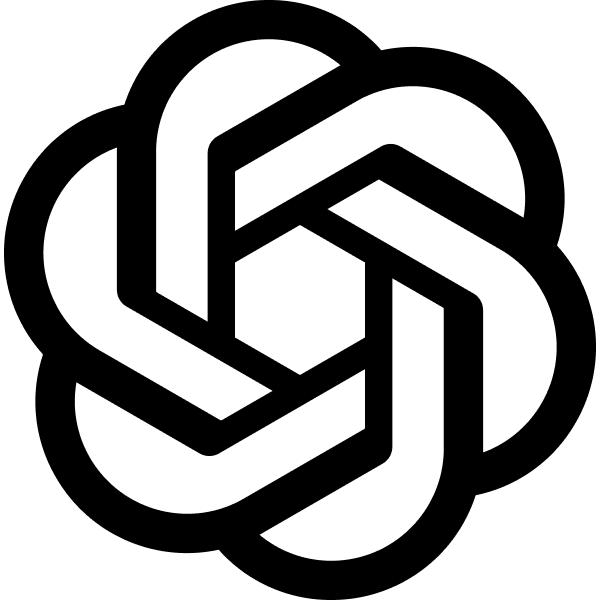
TODO: collapasable, copyable, download, etc
I have a Svelte component A that creates a writable store foo. It then passes foo as a prop to component B. In B's onMount, I do foo.subscribe
, but I get a console error "store.subscribe" is not a function. What is going on?The error message "store.subscribe is not a function" suggests that the foo
object you're trying to subscribe to in component B is not recognized as a Svelte store. This can happen due to a few reasons. Here's a checklist to help diagnose and fix the issue:
Ensure
foo
is indeed a writable store: In component A, make sure you're creatingfoo
using Svelte'swritable
function fromsvelte/store
. It should look something like this:import { writable } from 'svelte/store'; export let foo = writable(initialValue);
If
foo
is not created withwritable
(or another store function likereadable
orderived
), it won't have a.subscribe
method.Check the Prop Passing: Ensure that you're passing
foo
correctly as a prop to component B. In the parent component (A), you should have something like this:<!-- ComponentA.svelte --> <script> import ComponentB from './ComponentB.svelte'; import { writable } from 'svelte/store'; let foo = writable(someValue); </script> <ComponentB {foo}/>
Accessing the Store in Component B: In Component B, you should be able to subscribe to
foo
without any issue if it's properly passed as a prop. Here's an example of how it's typically done:<!-- ComponentB.svelte --> <script> import { onMount } from 'svelte'; export let foo; onMount(() => { const unsubscribe = foo.subscribe(value => { console.log(value); }); return () => { unsubscribe(); }; }); </script>
If
foo
is correctly received as a writable store, this should work without any error.Debugging Tip: Add a console log before the subscription to verify what
foo
looks like within Component B:onMount(() => { console.log(foo); // Check what foo is const unsubscribe = foo.subscribe(value => { console.log(value); }); // Rest of your code });
This can help you verify whether
foo
is what you expect it to be. Iffoo
logs asundefined
or as something other than a store object, then there's likely an issue with howfoo
is passed from Component A or how it's created.Component Compilation or Importing Issue: Make sure there are no compilation issues or errors in the way components are imported or used. Sometimes, the problem might be due to a misconfigured project setup or an overlooked error elsewhere in your code.
If you've gone through these steps and the problem persists, it might be helpful to review the component hierarchy and the data flow once more. Ensure that foo
is not inadvertently modified or reassigned to something that's not a store before being passed to Component B.
Related Chats
Update Svelte Store Item 0.632
Svelte Component Help 0.577
Client-Only Component in SvelteKit 0.550
Fix SvelteKit Playwright Test 0.521
Reactivity Issue Resolution 0.500
Store Iteration Order Issue 0.497
Svelte: Force New Component 0.494
JS Store Update Syntax 0.482