Dynamic Auto-Complete Component
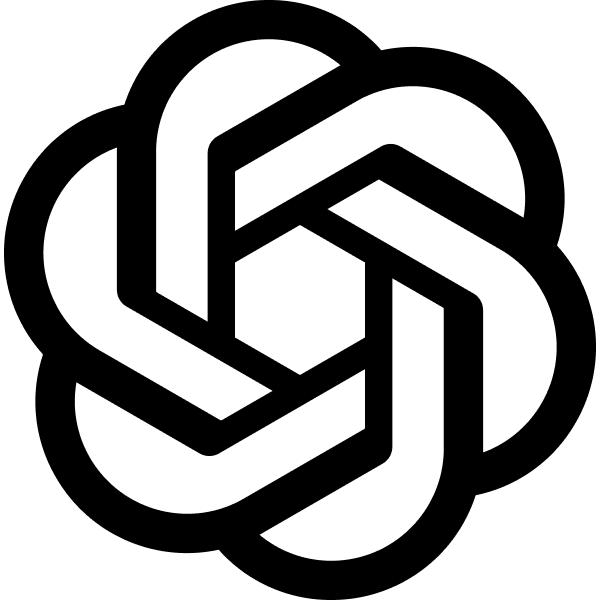
TODO: collapasable, copyable, download, etc
I have the following requirements.
I need to provide a variable options
to an Auto-complete component.
At first, options
will be a fixed set of data of filterKeys
.
The user can select be either typing to filter, pressing up and down arrows to move the current selector, and lastly pressing Enter to select... or they can simply click the item from a list.
Once the user selects a specific filterKey, then we will completely change the contents of options
.
The new contents will be what the user selected, say foo
, and then we expand all possible combinations of it with a list of filterOperators
such as ['=', '!=', 'contains', ...]
so options would be ['foo=', 'foo!=', 'foo contains', ...]
.
Again, the user can select from the options be either using the keyboard and Enter or by scrolling and clicking.
Also, when the user made the initial selection foo
, we use that as input to retrieve a valid set of values for foo,
such as ['bar', 'bazz', 'buzz', ...]
.
Initially, we just keep track of thes filterValues
, but once the user selects a second time, say they select foo=
,
We now update the options
with completely new values that are permutations of the selected foo=
and the filterValues, so
options is now ['foo=bar', 'foo=bazz', 'foo=buzz', ...]
.
What are your thoughts about implementing this in code?Your requirement describes a dynamic auto-complete component with multi-stage filtering and option generation. This implementation can be quite involved, but I'll outline a high-level approach that you can follow, using a combination of React and JavaScript (assuming a web-based UI).
Overview of Approach
Initial State: Initialize the component with a state that holds the
options
,selectedKey
,selectedOperator
, andfilterValues
.Rendering Options: Render the options based on the current state. Initially, it will display
filterKeys
.Handling Selections: When a user selects a
filterKey
, update the state to reflect the selected key and changeoptions
to show combinations withfilterOperators
.Fetching Filter Values: Once a
filterKey
is selected, fetch the correspondingfilterValues
asynchronously.Further Selections: When a user selects an operator, update the options to show permutations of the selected key-operator pair with the
filterValues
.Event Handling: Handle keyboard and mouse interactions for selection, navigation, and filtering within the options list.
Example in Code
Here's a simplified pseudo-code example using React:
import React, { useState, useEffect } from 'react';
const AutoCompleteComponent = ({ filterKeys, filterOperators }) => {
const [options, setOptions] = useState(filterKeys);
const [selectedKey, setSelectedKey] = useState(null);
const [selectedOperator, setSelectedOperator] = useState(null);
const [filterValues, setFilterValues] = useState([]);
// Fetch filter values based on the selected key
useEffect(() => {
if (selectedKey) {
fetchFilterValues(selectedKey).then(data => {
setFilterValues(data);
});
}
}, [selectedKey]);
const handleSelect = (selection) => {
if (!selectedKey) {
setSelectedKey(selection);
setOptions(filterOperators.map(op => `${selection}${op}`));
} else if (!selectedOperator) {
setSelectedOperator(selection);
setOptions(filterValues.map(value => `${selectedKey}${selection}${value}`));
}
// Additional logic for further selections if needed
};
// Render your options here
return (
<div>
{options.map((option, index) => (
<div key={index} onClick={() => handleSelect(option)}>
{option}
</div>
))}
</div>
);
};
// Mock function to simulate fetching filter values
const fetchFilterValues = async (key) => {
// Replace with actual fetch call
return ['bar', 'bazz', 'buzz'];
};
export default AutoCompleteComponent;
Considerations
Asynchronous Data Fetching: When fetching filter values, ensure that the asynchronous nature of the fetch is properly handled. Use
useEffect
for side effects like data fetching.UI/UX: Ensure that the component is user-friendly. Implement keyboard navigation and ensure that the list is scrollable and clickable.
Performance: For large sets of data, consider implementing lazy loading or virtual scrolling.
State Management: Depending on the complexity of your app, you might need more robust state management (like Redux).
Error Handling: Implement error handling for the data fetch and any other asynchronous operations.
Customization: Your component should be flexible enough to handle different types of
filterKeys
,filterOperators
, andfilterValues
.
This is a high-level guide. Depending on your exact requirements and tech stack, you may need to adjust this approach accordingly.This looks really good. I have the requirement that once selectedKey
has been selected, or even if selectedKey
and selectedOperator
have been selected... if the user starts hitting the backspace key, they should be able to "backup" to earlier states. So if we have the input foo=b
, but the user hits backspace once the input is foo=
, then we should
we should clear out selectedOperator
and reset the options
to ['foo=', 'foo!=', 'foo contains', ...]
.
If the user hits Backspace a second time, the the input is now foo
, we should should clear out selectedOperator
and reset the options
to ['foo', 'apple', 'banana', ...]
.
Is that possible? How would you structure the code?To implement the "backspace" feature that allows users to revert to earlier states, you'll need to modify the event handling logic in your auto-complete component. The key idea is to detect the backspace key event and update the component's state based on the current input value.
Here's how you can structure the code to achieve this:
Track User Input: Maintain a state for the user's current input. This will be different from the selected key or operator, as it represents the raw input in the text field.
Handle Backspace Event: When the backspace key is pressed, check the current input value and update the state accordingly.
Update Options Dynamically: Based on the new state after a backspace event, update the options to reflect the appropriate stage (filterKeys, filterOperators, filterValues).
Edge Cases: Ensure that the logic correctly handles edge cases, such as backspacing to an empty string.
Example Code Update
Here's an update to the previous example to include backspace handling:
import React, { useState, useEffect } from 'react';
const AutoCompleteComponent = ({ filterKeys, filterOperators }) => {
const [options, setOptions] = useState(filterKeys);
const [selectedKey, setSelectedKey] = useState(null);
const [selectedOperator, setSelectedOperator] = useState(null);
const [filterValues, setFilterValues] = useState([]);
const [inputValue, setInputValue] = useState('');
// Fetch filter values based on the selected key
useEffect(() => {
if (selectedKey) {
fetchFilterValues(selectedKey).then(data => {
setFilterValues(data);
});
}
}, [selectedKey]);
const handleSelect = (selection) => {
// Updated selection logic
};
const handleInputChange = (e) => {
const value = e.target.value;
setInputValue(value);
handleBackspace(value);
};
const handleBackspace = (value) => {
if (value.endsWith('=')) {
// If backspace leaves a key with '=', reset to operator selection
setSelectedOperator(null);
setOptions(filterOperators.map(op => `${selectedKey}${op}`));
} else if (!value.includes('=')) {
// If backspace leaves only a key, reset to key selection
setSelectedKey(null);
setSelectedOperator(null);
setOptions(filterKeys);
}
};
// Render your options and input field here
return (
<div>
<input value={inputValue} onChange={handleInputChange} />
{options.map((option, index) => (
<div key={index} onClick={() => handleSelect(option)}>
{option}
</div>
))}
</div>
);
};
// Other functions remain the same
export default AutoCompleteComponent;
Additional Considerations
Debounce Input Handling: If your input handling involves network requests or heavy computations, consider using a debounce mechanism to limit the frequency of these operations.
Clear and Reset States: Ensure that when you clear states (like
selectedOperator
), all dependent states and UI elements are also reset appropriately.User Experience: Provide clear visual feedback to the user when states are reset. This helps in understanding what's happening when they use backspace.
Testing: Thoroughly test the component with various input scenarios to ensure that the backspace logic works smoothly across different stages of selection.
This approach allows your component to dynamically respond to user input, making it flexible and user-friendly.Does handleBackspace need to know the previous input value as well as the current input value? This could tell us if the user is adding to their input or backing up, right?
=
here is just an example, this returning to earlier states should work for any of the filterOperators such as !=
and contains
as well
Yes, you're correct. To effectively determine whether the user is adding to their input or backspacing, it would be beneficial for handleBackspace
to know both the previous and the current input values. This comparison allows the function to discern the direction of the input change.
For handling different filter operators like !=
, contains
, etc., the logic needs to be more sophisticated. You would have to parse the current input string to identify the selected key and operator, and then decide how to revert to an earlier state based on this parsing.
Here's how you can modify the code to include these considerations:
Maintain Previous Input Value: Store the previous input value in the component's state to compare with the current input.
Enhanced Parsing Logic: Implement logic to parse the input string for different operators and handle backspace events accordingly.
Updated Code Example
import React, { useState, useEffect } from 'react';
const AutoCompleteComponent = ({ filterKeys, filterOperators }) => {
const [options, setOptions] = useState(filterKeys);
const [selectedKey, setSelectedKey] = useState(null);
const [selectedOperator, setSelectedOperator] = useState(null);
const [filterValues, setFilterValues] = useState([]);
const [inputValue, setInputValue] = useState('');
const [prevInputValue, setPrevInputValue] = useState('');
// Fetch filter values based on the selected key
useEffect(() => {
if (selectedKey) {
fetchFilterValues(selectedKey).then(data => {
setFilterValues(data);
});
}
}, [selectedKey]);
const handleSelect = (selection) => {
// Updated selection logic
};
const handleInputChange = (e) => {
const value = e.target.value;
setInputValue(value);
handleBackspace(prevInputValue, value);
setPrevInputValue(value);
};
const handleBackspace = (prevValue, currentValue) => {
if (isBackspacing(prevValue, currentValue)) {
// Logic to determine if the user is backspacing
// Parse currentValue and update state accordingly
}
};
const isBackspacing = (prevValue, currentValue) => {
return currentValue.length < prevValue.length;
};
// Render your options and input field here
// ...
};
// Other functions remain the same
export default AutoCompleteComponent;
Parsing Logic
The handleBackspace
function needs to parse the currentValue
to determine the state of the input. This involves checking whether the current input still contains the selected operator and if it matches the previously selected key. The complexity of this parsing will depend on the variety and format of your filter operators.
Additional Points
Robust Parsing: Depending on the complexity of your operators, you might need a more robust parsing strategy. Regular expressions or a small parsing utility might be helpful.
User Feedback: Inform the user when the state changes due to backspacing, possibly through UI cues or messages.
Testing: Thoroughly test with all operator types to ensure the logic works as expected across different scenarios.
This approach gives you the flexibility to handle a variety of input changes, including complex scenarios involving different filter operators.You're correct. To efficiently handle the backspace functionality and accommodate different filterOperators
, it's beneficial for the handleBackspace
function to be aware of both the current and the previous input values. This knowledge allows the function to determine whether the user is adding to their input or deleting (backspacing) it.
Moreover, to handle different operators like !=
, contains
, etc., the logic needs to be more generalized rather than specifically checking for the =
character.
Revised Approach:
Track Previous Input: Maintain a state to store the previous input value. Update this state whenever the input changes.
Generalize Operator Handling: Implement a more flexible method to determine the current state of the input (key selection, operator selection, value selection) that works with various operators.
Update Logic Based on Input Change: Use the comparison between the current and previous input values to determine if the user is adding or deleting characters, and update the state accordingly.
Example Code Update:
Here's an update to the code with these considerations:
import React, { useState, useEffect } from 'react';
const AutoCompleteComponent = ({ filterKeys, filterOperators }) => {
// ... other state declarations
const [inputValue, setInputValue] = useState('');
const [prevInputValue, setPrevInputValue] = useState('');
useEffect(() => {
// ... fetching filter values
}, [selectedKey]);
const handleInputChange = (e) => {
const value = e.target.value;
setPrevInputValue(inputValue);
setInputValue(value);
handleBackspace(value, prevInputValue);
};
const handleBackspace = (currentValue, previousValue) => {
// Check if user is backspacing
if (currentValue.length < previousValue.length) {
// Generalize the condition for different operators
if (filterOperators.some(op => currentValue.endsWith(op))) {
// Reset to operator selection
setSelectedOperator(null);
setOptions(filterOperators.map(op => `${selectedKey}${op}`));
} else if (!filterOperators.some(op => currentValue.includes(op))) {
// Reset to key selection
setSelectedKey(null);
setSelectedOperator(null);
setOptions(filterKeys);
}
}
};
// ... render method
};
// ... other functions remain the same
export default AutoCompleteComponent;
Additional Notes:
Handling Different Operators: The
some
method is used to check if any operator is currently at the end or is part of the input. This makes the function flexible for different types of operators.Efficient State Management: The comparison between
currentValue
andpreviousValue
is crucial to understand the user's action (adding vs. deleting).User Experience: Ensure that the behavior of the component is intuitive for users, especially when handling various operators.
By making these adjustments, your auto-complete component will be more robust and capable of handling a variety of user interactions smoothly.Will this logic work if we backspace enough to select a different fiterKey such as apple
? This is great, can you port this code to Svelete and output as complete of a Svelte component as possible? Thanks.The current implementation of the handleBackspace
function may not fully support the scenario where a user backspaces enough to change the filterKey
itself, such as switching from "foo" to "apple." This is because the logic is primarily focused on handling changes around the operators and not the filterKey
.
To handle this scenario, we need to extend the logic to detect changes in the filterKey
and update the state accordingly. This involves identifying when the input has changed significantly to represent a different key and then resetting the relevant states.
Extended Logic:
Determine Key Change: Detect when the current input value no longer corresponds to the previously selected
filterKey
.Reset States: If a different
filterKey
is detected, reset theselectedKey
,selectedOperator
, and other dependent states.Update Options: Update the options to reflect the new set of possible
filterKeys
or the appropriate state based on the new input.
Example Code Update:
Here's how you might update the code to handle changing the filterKey
:
const handleInputChange = (e) => {
const value = e.target.value;
setPrevInputValue(inputValue);
setInputValue(value);
handleBackspace(value, prevInputValue);
};
const handleBackspace = (currentValue, previousValue) => {
if (currentValue.length < previousValue.length) {
// Check if a different filterKey is being entered
if (!filterKeys.includes(currentValue) && !currentValue.includes('=')) {
// Reset to initial state with all filterKeys
setSelectedKey(null);
setSelectedOperator(null);
setOptions(filterKeys);
} else if (filterOperators.some(op => currentValue.endsWith(op))) {
// Reset to operator selection
setSelectedOperator(null);
setOptions(filterOperators.map(op => `${selectedKey}${op}`));
} else if (!filterOperators.some(op => currentValue.includes(op))) {
// Reset to key selection
setSelectedKey(null);
setSelectedOperator(null);
setOptions(filterKeys);
}
}
};
Additional Considerations:
Flexibility for Different Inputs: The logic should be flexible enough to handle various inputs and changes. It might need further refinement based on the specific format of your
filterKeys
andfilterOperators
.Performance: If your list of
filterKeys
orfilterOperators
is extensive, consider optimizing the checks to ensure the component remains responsive.User Feedback: Provide clear visual cues or feedback when the state changes significantly, like switching between different
filterKeys
.
By incorporating this extended logic, your component becomes more adaptable to user inputs, allowing for a dynamic and responsive user experience even when switching between different filterKeys
.Absolutely, I can help with converting the React component into a Svelte component. Svelte shares some similarities with React in terms of reactivity and component structure, but it has its own unique syntax and approach to state management and reactivity.
Here's how you might structure your auto-complete component in Svelte:
<script>
import { onMount } from 'svelte';
// Props (could be passed down from a parent component)
export let filterKeys = ['foo', 'apple', 'banana'];
export let filterOperators = ['=', '!=', 'contains'];
let options = filterKeys;
let selectedKey = null;
let selectedOperator = null;
let filterValues = [];
let inputValue = '';
let prevInputValue = '';
const fetchFilterValues = async (key) => {
// Replace with actual fetch call
return ['bar', 'bazz', 'buzz'];
};
const updateFilterValues = async () => {
if (selectedKey) {
filterValues = await fetchFilterValues(selectedKey);
}
};
onMount(updateFilterValues);
const handleSelect = (selection) => {
// ... selection logic
};
const handleInputChange = (event) => {
const value = event.target.value;
prevInputValue = inputValue;
inputValue = value;
handleBackspace(value, prevInputValue);
};
const handleBackspace = (currentValue, previousValue) => {
// ... backspace handling logic
};
</script>
<div>
<input bind:value={inputValue} on:input={handleInputChange} />
{#each options as option}
<div on:click={() => handleSelect(option)}>
{option}
</div>
{/each}
</div>
Key Points:
Reactivity: Svelte's reactivity is based on variable assignments. When you assign a new value to a variable, Svelte automatically updates the DOM.
Event Handling: Similar to React, but in Svelte, you use
on:eventName
to attach event listeners.Binding: The
bind:value
directive in Svelte creates a two-way binding on form elements. When the input changes,inputValue
is automatically updated and vice versa.Lifecycle: The
onMount
function is similar to React'suseEffect
with an empty dependency array. It runs once when the component is first rendered.Loops: The
{#each ...}
block is used to iterate over arrays.Async Functions: Just like in React, you can use async functions for fetching data.
Component Structure: In Svelte, the script, styling, and markup are typically contained within a single
.svelte
file.
Remember to tailor the logic inside handleSelect
and handleBackspace
to match the specific needs of your application. This example provides a structural foundation, but you'll need to fill in the details according to your application's requirements.
Related Chats
Svelecte Auto-Complete Behavior 0.532
Value Prop Reactivity Issue 0.523
Reactivity Troubleshooting in Svelte 0.477
Fix ExpandableGroupPanel Filter Error 0.466
Dropdown Button Component 0.413
Dynamic Filter Component 0.402
Svelte: Force New Component 0.393
Work Update - Jan 11 0.389