Merge and Deduplicate Tailwind Classes
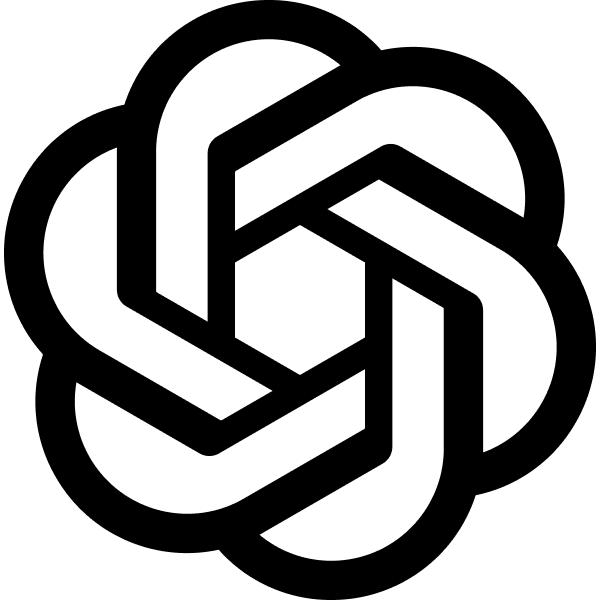
TODO: collapasable, copyable, download, etc
I have a react component that wraps a Flowbite React component. I want to pass through a className
of tailwind classes, so that users of my component can custommize it. Is there a way to detect and eliminate duplicate tailwind classes that do the same thing? For example if I am called with w-full
, then I don't want to show my w-80
that I would use otherwise. I can use a confitional check, but does tailwind provide an API to merge class lists?Tailwind CSS itself doesn't provide an API to programmatically merge or deduplicate class names based on their functionality or effect. This means that you can't directly ask Tailwind to figure out that w-full
should override w-80
, as this kind of logic is not built into Tailwind CSS at a utility level.
However, you can manage this situation in your React component by implementing a custom function to handle class name merging and deduplication. The idea is to prioritize user-provided classes over default ones, especially when they conflict in terms of functionality. For classes that directly conflict (like width, padding, margin, etc.), you can set up a system where user-defined classes override the default ones.
Here’s a simple approach using a custom function to handle potential conflicts by defining a priority list for conflicting classes:
- Define a list of conflicting class groups. Each group contains classes that should not coexist.
- When merging classes, check if any class from the user's input conflicts with the default classes.
- Remove the lower priority class from the final class list.
This example doesn't cover all cases and is tailored for width classes (w-*
), but you can extend it to other conflicting class types:
const mergeClassNames = (defaultClasses, userClasses) => {
// Define groups of classes that are in conflict with each other.
const conflictGroups = [
new Set(['w-full', 'w-1/2', 'w-1/3', 'w-1/4', 'w-1/5', 'w-80']),
// Add other groups as needed.
];
const userClassArray = userClasses.split(' ');
const defaultClassArray = defaultClasses.split(' ');
// Filter out default classes that conflict with user classes
const filteredDefaultClasses = defaultClassArray.filter((defaultClass) => {
return !conflictGroups.some((group) => {
return group.has(defaultClass) && userClassArray.some(userClass => group.has(userClass));
});
});
// Combine user classes with filtered default classes
return [...new Set([...filteredDefaultClasses, ...userClassArray])].join(' ');
};
// Usage
const YourComponentWrapper = ({ className, ...props }) => {
const defaultClassName = "w-80 p-4"; // Default Tailwind classes
const finalClassName = mergeClassNames(defaultClassName, className);
// Pass finalClassName to the Flowbite component or another element
return <YourFlowbiteComponent className={finalClassName} {...props} />;
};
This function splits the default and user class names into arrays, checks for conflicts, and removes the conflicting default classes if a user class from the same conflict group is present. It finally merges the remaining default classes with the user classes, deduplicates them, and returns the result. This approach can be expanded to include more conflict groups and tailored to your specific needs.
Merge and Deduplicate Tailwind Classes created at Tue Jan 20 1970 19:13:12 GMT+0000 (Coordinated Universal Time)Related Chats
Merge and Deduplicate Tailwind Classes 1.000
Conditional UserProvider Wrapper 0.395
React TypeScript - Handle Unknown 0.372
React TypeScript Component: Props & Render 0.366
Find parent with class. 0.357
Tailwind CSS 11px Update 0.334
React Auth Types Enum 0.327
Use Effect vs Component Body 0.312
Listen for Command+K. 0.306
Dismiss Pop-up on Click 0.305