React TypeScript Component: Props & Render
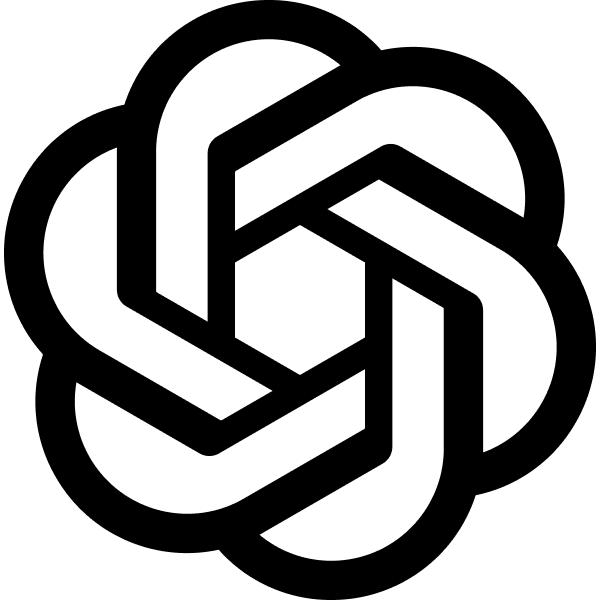
TODO: collapasable, copyable, download, etc
I am writing a Rect funcational component in Typescript that should take the following props - size - string (one of "xs", "sm", "md", "lg") and default to "md" if none are present. color ("blue", "purple", "gray" etc and default to "blue"), and the body uses the children to render whatever is in side the element. Can you give me that code with a simple
<div>
element that uses the provided size
and color
props, defaulting to "md" and "blue" respectively if none are provided. The component also renders its children within the <div>
.
import React, { ReactNode } from 'react';
interface MyComponentProps {
size?: 'xs' | 'sm' | 'md' | 'lg';
color?: 'blue' | 'purple' | 'gray';
children?: ReactNode;
}
const MyComponent: React.FC<MyComponentProps> = ({
size = 'md',
color = 'blue',
children,
}) => {
// Example of how you might use the size and color for styling or class names
const className = `component-${size} component-${color}`;
// Simple implementation of the div with dynamic class names
return (
<div className={className}>
{children}
</div>
);
};
export default MyComponent;
In this example, the className
is constructed using the size
and color
props to demonstrate how you might use these props for styling purposes. In a real scenario, you would likely have CSS classes corresponding to each size
and color
value to apply the desired styles to the component. The children
prop is used to render whatever content is passed within the component when it is used.
Here's a quick example of how you might use this component:
<MyComponent size="lg" color="purple">
<p>This is some content inside the component.</p>
</MyComponent>
This would render a <div>
with class names corresponding to "large" and "purple" containing a paragraph of text.How would I put a key on this? {chatHistory.map((message: ChatMessage) => { switch (message.type) { case ChatMessageType.USER_CHAT_MESSAGE: return <ChatTextMessage message={message} />; case ChatMessageType.SYSTEM_RESULTS_KEY: return <SystemResponseMessage message={message} />; } })}
Related Chats
React TypeScript - Handle Unknown 0.623
Use Effect vs Component Body 0.593
Conditional UserProvider Wrapper 0.572
React JSX Conditional Rendering 0.515
No need setFoo. 0.490
Svelte: Force New Component 0.486
Listen for Command+K. 0.464
React Auth Types Enum 0.444