React TypeScript - Handle Unknown
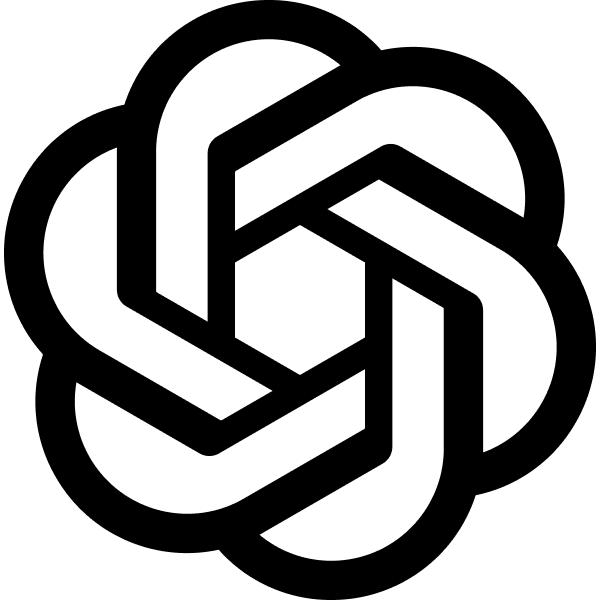
TODO: collapasable, copyable, download, etc
React Typescript is giving me this error. ```./src/app/components/DAISidebarBottom.tsx:57:15 Type error: Type 'unknown' is not assignable to type 'ReactNode'.
55 |
57 | {user?.given_name}```
I can fix it with this <div className="text-sm font-medium dark:text-gray-100"> {user?.given_name ? String(user?.given_name) : ''} </div>
But... is there a more elegant or correct way to fix this?The error you're encountering in your TypeScript React project is a common issue related to type safety when handling data that could potentially be of an unknown or undefined type. TypeScript is strict about ensuring that values used in your JSX elements are compatible with React's type definitions, where ReactNode
includes string
, number
, boolean
, ReactElement
, etc., but not unknown
or undefined
.
Your current solution effectively handles the case where user?.given_name
might be undefined or have a type that isn't directly compatible with ReactNode
by explicitly converting it to a string, or defaulting to an empty string if it's not present. This is perfectly valid and works well. However, if you're looking for a more concise or potentially more "elegant" approach, you can consider a few alternatives:
1. Implicit Conversion
You can leverage JavaScript's coercion to convert any value to a string more succinctly:
<div className="text-sm font-medium dark:text-gray-100">
{String(user?.given_name)}
</div>
This will convert undefined
to "undefined"
, which might not be desirable. If you prefer to display nothing when given_name
is undefined, your original solution with a ternary operator or the ||
operator is better.
2. Using Logical OR for Defaults
If you want to avoid displaying "undefined"
or other falsy values, you can use:
<div className="text-sm font-medium dark:text-gray-100">
{user?.given_name || ''}
</div>
This will display the name if it exists, or an empty string otherwise. This is very similar to your solution but uses logical OR, which is a bit more streamlined.
3. Type Assertion
If you are absolutely sure user?.given_name
will always be a string, you could use type assertion:
<div className="text-sm font-medium dark:text-gray-100">
{user?.given_name as string}
</div>
This tells TypeScript you are certain about the type, though it's not safe if there's any chance given_name
might not be a string.
4. Providing a Default Value on Data Fetching
Another approach could involve ensuring that user?.given_name
is always a string when the user object is fetched or constructed, thus avoiding this issue in the component entirely:
// When fetching or constructing the user object
const user = {
given_name: fetchedUser.given_name || ''
};
This way, you handle the default value upstream, and the component remains clean and focused only on presentation.
Each of these methods has its place depending on how much control you have over the data and your specific needs regarding handling falsy or undefined values. Your original solution is actually quite good in terms of explicitness and safety.
Related Chats
React TypeScript - Handle Unknown 0.999
React TypeScript Component: Props & Render 0.623
Conditional UserProvider Wrapper 0.572
TS Default Value Fix 0.562
React JSX Conditional Rendering 0.479
React Auth Types Enum 0.453
Type Chats: ChatSession Creation 0.422
SSR Error: HTMLElement Not Defined 0.418