Stress Testing Rust Bidding
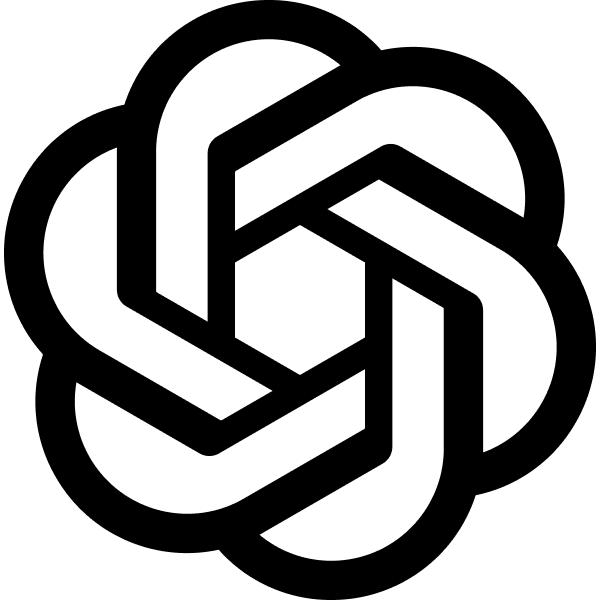
TODO: collapasable, copyable, download, etc
I have some Rust code to testing bidding. I want to stress test the following:
- the first bid that meets business rules (bid is at least $1 more than current winning bid, etc) should be accepted as the new current winning bid
- that other simultaneous bids are rejected
How would you structure and write this Rust test?To stress test the bidding system in Rust, you'd want to simulate multiple threads submitting bids simultaneously to see if the system adheres to the requirements you mentioned.
Let's break down the task:
Bidding Logic: First, you need a basic representation of your bidding system. For simplicity, let's assume there's a shared
BidManager
that handles all bid submissions.Multi-threaded Bidding: Use the
std::thread
module in Rust to create multiple threads that will submit bids simultaneously.Testing the Conditions: After all bids are submitted, ensure only the first valid bid is accepted and all others are rejected.
Here's a basic test structure to achieve this:
use std::sync::{Arc, Mutex};
use std::thread;
const START_BID: i32 = 100;
struct BidManager {
current_bid: Mutex<i32>,
}
impl BidManager {
fn new(start_bid: i32) -> Self {
BidManager {
current_bid: Mutex::new(start_bid),
}
}
fn submit_bid(&self, bid: i32) -> bool {
let mut current_bid = self.current_bid.lock().unwrap();
if bid > *current_bid + 1 {
*current_bid = bid;
true
} else {
false
}
}
}
#[test]
fn stress_test_bidding() {
let bid_manager = Arc::new(BidManager::new(START_BID));
let mut handles = vec![];
for i in 0..10 {
let bid_manager_clone = bid_manager.clone();
let handle = thread::spawn(move || {
bid_manager_clone.submit_bid(START_BID + i)
});
handles.push(handle);
}
let mut accepted_count = 0;
for handle in handles {
if handle.join().unwrap() {
accepted_count += 1;
}
}
// Only one bid should be accepted.
assert_eq!(accepted_count, 1);
}
This is a basic version that will submit bids simultaneously from 10 threads. Each bid is only one dollar more than the previous bid, so only the first valid bid should be accepted.
You can expand upon this structure and make it more sophisticated, such as adding more complex bid submissions, handling potential race conditions more explicitly, or incorporating more of your business rules. However, this should give you a good starting point for a stress test.How can I set a Rust env variableIn Rust, you can set environment variables for the process using the std::env
module. Specifically, you can use the set_var
function.
Here's how you can set an environment variable:
use std::env;
fn main() {
env::set_var("MY_VARIABLE", "my_value");
// To check that the variable was indeed set
assert_eq!(env::var("MY_VARIABLE").unwrap(), "my_value");
println!("MY_VARIABLE = {}", env::var("MY_VARIABLE").unwrap());
}
Note that this sets the environment variable for the current process. If you run another program from this one using something like std::process::Command
, the child process will inherit this environment variable. However, this does not set the environment variable globally or for other processes not spawned by this one.
If you meant setting environment variables for the build process via Cargo
, you can do so outside the Rust code, in the shell or command prompt:
export MY_VARIABLE=my_value
cargo run
Or you can do it inline with the cargo run
command:
MY_VARIABLE=my_value cargo run
Remember that the behavior and syntax might differ a bit between platforms, like Windows and Unix-based systems.Should I use a db transaction and check different business logic in Rust code, or should I try to implement all my business logic in SQL for checking if a bid is allowed and to insert into two tables, the max_bids table and the bid_history table?Whether you should implement business logic in Rust code or in SQL heavily depends on your specific use case, the complexity of your application, and your long-term maintenance goals. Here are some pros and cons for each approach:
Business Logic in Rust Code:
Pros:
- Readability and Maintenance: For developers familiar with Rust but not with the intricacies of SQL, especially complex business logic, it might be easier to read and maintain the code.
- Flexibility: Rust provides powerful type-checking, error handling, and abstraction capabilities. Implementing complex business logic might be simpler and safer in Rust.
- Portability: If you decide to change your database system, having the business logic in Rust might mean fewer changes, as the main logic remains in the Rust layer.
Cons:
- Performance: Multiple trips to the database (e.g., one for checking and another for inserting) might introduce latency.
- Concurrency: Without using database transactions, you risk running into race conditions where, for instance, two bids might get accepted when only one should.
Business Logic in SQL:
Pros:
- Performance: Implementing logic directly on the database might be faster because it reduces the number of database trips. For instance, you can check a condition and make an insert in a single transaction.
- Atomicity: SQL transactions ensure that all commands succeed or fail together, which can be beneficial for maintaining data consistency.
- Data Integrity: Databases offer constraints like unique, check, and foreign key constraints which can be used to enforce certain business rules at the data layer.
Cons:
- Complexity: SQL, especially when mixed with stored procedures and triggers for complex logic, can become hard to read and maintain.
- Portability: Specific SQL logic, especially if it uses features unique to a particular DBMS, can make your application tightly coupled to that DBMS.
- Testing: It might be harder to test complex SQL logic compared to Rust code.
Recommendation:
- Combined Approach: Use database transactions in conjunction with Rust for best results. Start a transaction in Rust, apply necessary checks in Rust, interact with the database, and commit or roll back the transaction based on the results. This approach combines the flexibility and readability of Rust with the atomicity and performance benefits of database transactions.
- Use Simple SQL for Data Integrity: Use SQL constraints for data integrity checks that are straightforward and fit well with the relational model.
- Complex Business Logic in Rust: If the business logic is complex, it might be more maintainable and testable in Rust.
Always consider the long-term maintainability, team's expertise, and specific requirements of your application when making this decision.I am trying to create a way to pass around the db transaction.
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let mut conn = Connection::open(&db_url)?;
let tx = conn.transaction()?;
Ok(tx)
}```
Has the error ```error[E0515]: cannot return value referencing local variable `conn`
--> src\model\auction_bidding_client.rs:11:5
|
10 | let tx = conn.transaction()?;
| ------------------ `conn` is borrowed here
11 | Ok(tx)
| ^^^^^^ returns a value referencing data owned by the current function```
How do I fix this?The error you're encountering arises from Rust's ownership system. The problem is that the `transaction()` method borrows `conn`, and when you try to return `tx`, you're effectively trying to return a reference to something (`conn`) that's going out of scope. Once the function ends, `conn` will be dropped, but `tx` still refers to it, which is disallowed by Rust to prevent accessing dangling references.
To fix this, you'll need to ensure that both the `Transaction` and the `Connection` outlive the function. This is typically achieved by wrapping them together in some sort of struct that owns both.
Here's an approach using a struct:
```rust
use rusqlite::{Connection, Transaction};
use std::env;
pub struct TransactionContext {
pub conn: Connection,
pub tx: Transaction<'static>,
}
impl TransactionContext {
pub fn new() -> anyhow::Result<Self> {
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
let tx = conn.transaction()?;
Ok(Self { conn, tx })
}
}
// Usage
pub fn some_function() -> anyhow::Result<()> {
let mut context = TransactionContext::new()?;
// Use context.tx for your transaction operations
Ok(())
}
With the above approach:
TransactionContext
owns both the connection (conn
) and the transaction (tx
).- When
TransactionContext
goes out of scope, bothconn
andtx
will be dropped safely.
This pattern ensures that the Connection
and the Transaction
have the same lifetime and avoids the ownership issues you encountered.Should TransactionContext code live with the caller code or here in the db client code?The decision on where to place the TransactionContext
struct, whether within the database client code or with the caller, depends on several factors. Here are some guidelines to help you decide:
TransactionContext in the DB Client Code:
Pros:
- Encapsulation: By putting
TransactionContext
in the database client code, you encapsulate the details of transaction management and keep it separate from the business logic in the caller. This keeps your database-related code modular and self-contained. - Reuse: If multiple parts of your application need transactional operations, having the
TransactionContext
within the DB client code allows for easy reuse without duplication. - Consistency: Ensures that all transactions are handled consistently across different parts of your application.
Cons:
- Complexity: If the transaction management logic is not simple or needs to be customized often, placing it within the DB client might make the client code more complex.
TransactionContext in the Caller Code:
Pros:
- Flexibility: By having the
TransactionContext
in the caller code, it's easier to customize its behavior for specific use cases without affecting other parts of the application. - Explicit Control: The caller has more explicit control over transaction lifetimes and can better decide when to start, commit, or rollback.
Cons:
- Duplication: If multiple parts of your application require transactional operations, you might end up duplicating the transaction context creation logic.
- Less Encapsulation: Spreading database transaction logic throughout your application can make the code harder to maintain.
Recommendation:
In most cases, if the TransactionContext
is meant to be a general utility for handling database transactions, it's better to have it in the DB client code. This approach centralizes transaction management, making it easier to maintain and update in the future.
However, if there's a very specific use case where the transaction handling is unique and not reusable, then it might make sense to have it in the caller code.
Remember, it's essential to strike a balance between encapsulation and flexibility. It's generally a good practice to encapsulate database-related operations in one module or layer to ensure a clean separation of concerns.I tried this code ```impl TransactionContext { pub fn new() -> anyhow::Result { let db_url = env::var("AUCTION_BIDDING_DB_URL")?; let mut conn = Connection::open(&db_url)?; let tx = conn.transaction()?; Ok(Self { conn, tx }) } }
pub fn create_transaction() -> anyhow::Result { let mut context = TransactionContext::new()?; Ok(context) }```
It has this error ```error[E0597]: conn
does not live long enough
--> src\model\auction_bidding_client.rs:16:18
|
15 | let mut conn = Connection::open(&db_url)?;
| -------- binding conn
declared here
16 | let tx = conn.transaction()?;
| ^^^^^^^^^^^^^^^^^^
| |
| borrowed value does not live long enough
| argument requires that conn
is borrowed for 'static
17 | Ok(Self { conn, tx })
18 | }
| - conn
dropped here while still borrowed
error[E0505]: cannot move out of conn
because it is borrowed
--> src\model\auction_bidding_client.rs:17:19
|
15 | let mut conn = Connection::open(&db_url)?;
| -------- binding conn
declared here
16 | let tx = conn.transaction()?;
| ------------------
| |
| borrow of conn
occurs here
| argument requires that conn
is borrowed for 'static
17 | Ok(Self { conn, tx })
| ^^^^ move out of conn
occurs here```
How can I fix this?The errors you're encountering are due to lifetimes associated with the transaction and the connection.
When you call conn.transaction()?
, a transaction (tx
) is created which holds a reference to the connection (conn
). As a result, you can't move conn
into the TransactionContext
while also holding a reference to it in tx
.
To address this, you should modify the design slightly. Instead of having tx
be a field in TransactionContext
, it's better to store the connection itself and only create the transaction when needed.
Here's a modified version:
use rusqlite::Connection;
use std::env;
pub struct TransactionContext {
pub conn: Connection,
}
impl TransactionContext {
pub fn new() -> anyhow::Result<Self> {
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
Ok(Self { conn })
}
// This method can be called when you actually need the transaction
pub fn transaction(&self) -> anyhow::Result<rusqlite::Transaction> {
self.conn.transaction()
}
}
pub fn create_transaction() -> anyhow::Result<TransactionContext> {
let context = TransactionContext::new()?;
Ok(context)
}
With this design, the TransactionContext
only contains the connection. You then use the transaction
method on the TransactionContext
to get a transaction when you need it. This separation ensures that the lifetime of the transaction is tied to a specific method call, rather than trying to store both the connection and the transaction in the struct at the same time, which leads to the lifetime conflicts you observed.Now I am trying to use my new transaction tx
variable.
let max_bids = get_all_max_bids(&mut tx, auction_id, lot_number)?;
```
and I updated the db code
```pub fn get_all_max_bids(tx: &mut Transaction, auction_id: u32, lot_number: u32) -> anyhow::Result<Vec<MaxBid>> {
let mut max_bids = Vec::new();
let auction_lot_id = format!("a{auction_id}-l{lot_number}");
//let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
//let conn = Connection::open(&db_url)?;
let mut stmt = tx.prepare(
r#"
...```
But I get this error ```error[E0308]: mismatched types
--> src\service\auction\bidding.rs:64:37
|
64 | let max_bids = get_all_max_bids(&mut tx, auction_id, lot_number)?;
| ---------------- ^^^^^^^ expected `&mut Transaction<'_>`, found `&mut TransactionContext`
| |
| arguments to this function are incorrect
|
= note: expected mutable reference `&mut Transaction<'_>`
found mutable reference `&mut TransactionContext`
note: function defined here
--> src\model\auction_bidding_client.rs:29:8
|
29 | pub fn get_all_max_bids(tx: &mut Transaction, auction_id: u32, lot_number: u32) -> anyhow::Result<Vec<MaxBi...
| ^^^^^^^^^^^^^^^^ --------------------```
How do I fix this?The error you're seeing is because you're trying to pass a `TransactionContext` to `get_all_max_bids()`, but the function expects a `Transaction`.
To resolve the issue, you should first get the `Transaction` from the `TransactionContext` and then pass it to `get_all_max_bids()`.
Here's how you can do it:
1. In your service code, get the transaction from the `TransactionContext`:
```rust
let mut context = create_transaction()?;
let mut tx = context.transaction()?;
let max_bids = get_all_max_bids(&mut tx, auction_id, lot_number)?;
- Make sure the
get_all_max_bids()
function expects aTransaction
:
pub fn get_all_max_bids(tx: &mut rusqlite::Transaction, auction_id: u32, lot_number: u32) -> anyhow::Result<Vec<MaxBid>> {
// ... rest of the code
}
This way, you're separating the transaction creation from its use, which gives you a clearer separation of concerns and avoids lifetime and type mismatches.I am trying to handle either Conn or Transaction.
Some(tx) => tx.prepare(sql)?,
None => {
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
let x = conn.prepare(sql)?;
x
}
};```
But I get this error ```error[E0597]: `conn` does not live long enough
--> src\model\auction_bidding_client.rs:110:21
|
105 | let mut stmt = match tx {
| -------- borrow later stored here
...
109 | let conn = Connection::open(&db_url)?;
| ---- binding `conn` declared here
110 | let x = conn.prepare(sql)?;
| ^^^^^^^^^^^^^^^^^ borrowed value does not live long enough
111 | x
112 | }
| - `conn` dropped here while still borrowed```The issue you're encountering is because you're trying to return a prepared statement (`x` in your code) that borrows from a connection (`conn`), but the connection is dropped at the end of the `None` branch, making the prepared statement a dangling reference.
The `prepare` method returns a `Statement`, which borrows from the `Connection`. When you create the `Statement` inside the `match` block and try to return it, it still holds a reference to the `Connection`, which is dropped immediately after the block, causing the error.
To resolve this, you have a few options:
1. **Always use a Transaction**: If possible, always pass in a transaction. This ensures consistent behavior and avoids the need to handle two cases.
2. **Refactor to avoid borrowing**: Instead of returning the statement, execute your database operations inside the match branches and return the result.
Here's an example of the second approach:
```rust
fn some_db_function(tx: Option<&mut rusqlite::Transaction>, sql: &str) -> anyhow::Result<()> {
match tx {
Some(tx) => {
let mut stmt = tx.prepare(sql)?;
// ... execute your operations on the statement ...
},
None => {
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
let mut stmt = conn.prepare(sql)?;
// ... execute your operations on the statement ...
}
}
Ok(())
}
With this approach, you handle the database operations directly within the match branches, ensuring that any borrows remain valid for their entire scope.
If you still need the flexibility of handling both Connection
and Transaction
, and you want to perform the same operation for both, consider using a trait or similar mechanism to abstract away the differences between them. However, for simplicity, the approach above should suffice in most cases.let tx = match tx { Some(tx) => tx, None => { let db_url = env::var("AUCTION_BIDDING_DB_URL")?; let mut conn = Connection::open(&db_url)?; let tx = &mut conn.transaction()?; tx } };
has the error ```error[E0597]: conn
does not live long enough
--> src\model\auction_bidding_client.rs:102:27
|
90 | tx: Option<&mut Transaction>,
| -- has type std::option::Option<&mut Transaction<'1>>
...
101 | let mut conn = Connection::open(&db_url)?;
| -------- binding conn
declared here
102 | let tx = &mut conn.transaction()?;
| ^^^^^^^^^^^^^^^^^^-
| |
| borrowed value does not live long enough
| assignment requires that conn
is borrowed for '1
103 | tx
104 | }
| - conn
dropped here while still borrowed
error[E0716]: temporary value dropped while borrowed
--> src\model\auction_bidding_client.rs:102:27
|
102 | let tx = &mut conn.transaction()?;
| ^^^^^^^^^^^^^^^^^^^ creates a temporary value which is freed while still in use
103 | tx
| -- borrow later used here
104 | }
| - temporary value is freed at the end of this statement
|
= note: consider using a let
binding to create a longer lived value```You're facing a common problem with lifetimes and ownership in Rust. The error occurs because you're trying to return a reference to a Transaction
created from a Connection
that's local to the match
block. When the block ends, the Connection
(conn
) will be dropped, and hence any reference to a Transaction
created from it will be invalid.
The simplest solution is to always use a Transaction
, either passed in or created anew, and ensure the ownership of the underlying connection is maintained for the life of the transaction. In practice, this requires some restructuring of your code.
Here's an outline of what you might do:
- Use an enum to represent either a borrowed transaction or an owned connection+transaction pair.
- Implement the necessary logic on that enum.
Here's a possible implementation:
use rusqlite::{Connection, Transaction};
enum TransactionOrConn {
Borrowed(Transaction<'static>),
Owned(Connection, Transaction<'static>),
}
impl TransactionOrConn {
fn new(tx: Option<Transaction<'static>>) -> anyhow::Result<Self> {
match tx {
Some(tx) => Ok(Self::Borrowed(tx)),
None => {
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
let tx = conn.transaction()?;
Ok(Self::Owned(conn, tx))
}
}
}
// For any operation you want to execute, you can implement a method on this enum
// and internally match to decide which variant to use.
fn some_db_operation(&self, sql: &str) -> anyhow::Result<()> {
match self {
Self::Borrowed(tx) => {
// use tx to execute sql
}
Self::Owned(_, tx) => {
// use tx to execute sql
}
}
Ok(())
}
}
// Usage:
let tx_or_conn = TransactionOrConn::new(tx_option)?;
tx_or_conn.some_db_operation("YOUR SQL HERE")?;
With this approach:
- If you're given an existing transaction, it's used directly.
- If you need to create a new transaction, you also maintain ownership of the connection for as long as the transaction exists.
By wrapping everything inside the TransactionOrConn
enum, you ensure all lifetimes and ownerships are properly managed.When I run our threads, I get Error Code 5 database is locked errors. If I add tx.busy_timeout(Durection::from_seconds(5))
, I expected this to fix it, but I still get those errors. Shouldn't busy_timeout
cause SQLite library to keep trying to open the file for up to 5 seconds?You're correct in understanding that busy_timeout
sets a busy timeout on the database connection. If an operation is unable to proceed because of a locked database, it will keep retrying for the specified duration. However, there are a few reasons why you might still encounter the "database is locked" error:
Long-Running Transactions: If any of your threads or processes hold a transaction open for a duration longer than the timeout, you'll still encounter this error. Make sure to commit or rollback transactions promptly to free up the database for other threads or processes.
Journal Mode: SQLite uses a rollback journal to implement transactions. Depending on the journal mode, you might get locks. The
WAL
(Write-Ahead Logging) mode can be more concurrent than the default mode, so switching to it might help. You can enable WAL mode with the following SQL command:PRAGMA journal_mode=WAL;
.External Access: If any external process (not part of your multi-threaded application) accesses the database, it can lock it. Ensure no external tools or applications are accessing the database while your application is running.
Connection and Transaction Management: Ensure that you manage connections and transactions properly. For example, if you have a global connection or if threads don't clean up transactions properly, you can run into locking issues.
Timeout Duration: Even if you've set a timeout of 5 seconds, it might still not be long enough, depending on your workload. You could try increasing the timeout, but this would be more of a workaround than a solution. It's generally better to find the root cause of the contention and address it.
SQLite Limitations: SQLite, being a serverless, self-contained database, has some concurrency limitations compared to client-server databases. If your application has heavy concurrency requirements, you might hit these limits. If that's the case, consider using a more robust client-server database system, or architecting your application to minimize concurrent database access.
Busy Handler vs Timeout: While you're using
busy_timeout
, SQLite also provides a more granular way to handle busy situations using theset_busy_handler
method, which allows you to define a custom handler function that's invoked when SQLite encounters a busy situation.
To further diagnose the problem:
- Identify which operations are causing the lock. Is it during a read, write, or both?
- Determine if there's a pattern to when the locks happen. Is it immediately upon startup, after some runtime, or during specific operations?
- Monitor how long transactions are held open.
After gathering this information, you can adjust your application's behavior, connection settings, or database configuration accordingly.I am commit or rollback on the transaction. This code runs very quickly in less than 1 secondIf your code runs quickly and you are properly committing or rolling back transactions, then it sounds like you have contention on the database, possibly from multiple threads or tasks attempting to access the database simultaneously.
Here are some steps to troubleshoot and potentially resolve the issue:
WAL Mode: Ensure you're using the Write-Ahead Logging (WAL) journal mode, as mentioned earlier. This mode is more concurrent than the default mode and can significantly reduce the "database is locked" errors.
conn.execute("PRAGMA journal_mode=WAL;", [])?;
Thread Safety: Ensure that each thread has its own SQLite connection. Sharing SQLite connections across threads can lead to locking issues.
Limited Write Operations: SQLite supports multiple concurrent readers but only one writer at a time. If you have a lot of write operations, they might be waiting for the lock and exceed the timeout. In that case, consider:
- Batching Writes: Instead of many small writes, batch them together in fewer transactions.
- Off-Peak Writing: If possible, schedule intensive write operations during periods of low read activity.
Avoid Long-Running Read Transactions: Even if your write transactions are fast and promptly committed, a long-running read transaction can prevent writes from going through. Make sure that you also promptly finish any read transactions.
Check for Nested Transactions: Ensure that there are no nested transactions. Nested transactions can inadvertently hold locks longer than expected.
Connection Pooling: If you're creating and destroying connections rapidly, consider using a connection pool. This can help manage connections more efficiently and avoid potential contention when creating/destroying connections.
Logging & Monitoring: Add logging to your application to monitor when transactions begin, commit, and rollback. This might help you identify any unexpected behavior or contention points.
Locking Mode: You can try setting the SQLite locking mode to exclusive which might help in certain use cases. But be aware that this will prevent other database connections until the lock is released:
conn.execute("PRAGMA locking_mode=EXCLUSIVE;", [])?;
Foreign Key Checks: If you're using foreign key constraints, SQLite will have to do additional checks when inserting/updating/deleting data. You can temporarily defer these checks, but remember to re-enable them and manually verify the constraints before committing:
conn.execute("PRAGMA foreign_keys=OFF;", [])?; // Do your operations conn.execute("PRAGMA foreign_keys=ON;", [])?;
Disk I/O: Even though the code runs quickly, disk I/O, especially if the database is located on a network drive or slow storage, can affect performance. Ensure that the storage medium where the SQLite database resides has fast read/write speeds.
Finally, if you continue to experience issues and have implemented the above suggestions, it might be worthwhile to reconsider if SQLite is the right tool for the job. SQLite is fantastic for many applications, but there are situations, especially under high concurrency, where client-server databases like PostgreSQL, MySQL, or others might be more appropriate.Do I use conn.execute("PRAGMA journal_mode=WAL;", [])?;
everywhere I setup a connection?Yes, if you've decided to use the Write-Ahead Logging (WAL) journal mode for SQLite (which can help with concurrency issues), you should set it for every new SQLite connection you establish. Once it's set for a particular database file, it will persist across connections, but setting it for every new connection ensures that you always have it enabled, especially if the database could be used by other tools or systems that might change the mode.
Typically, you'd do this right after establishing a new connection:
let db_url = env::var("AUCTION_BIDDING_DB_URL")?;
let conn = Connection::open(&db_url)?;
// Enable WAL mode
conn.execute("PRAGMA journal_mode=WAL;", [])?;
In case you have a central place or function where you establish database connections, you can ensure the PRAGMA statement is executed there, so you don't have to remember to include it every time you create a connection elsewhere in your code.
Remember that, while WAL can help with concurrency issues, it's also slightly different in terms of behavior and disk usage. For instance, there are "checkpoint" operations that you might need to be aware of, and WAL can use additional disk space for its log file (-wal
file alongside your main database file). Be sure to read up on WAL mode to understand its characteristics fully.
Related Chats
Stress Testing Rust Bidding 1.000
Tests untuk initializeNewThread 0.440
Nested vec! Macro Usage 0.419
Rust Enum for Strings 0.412
Rust Marketplace Scraper. 0.402
Rust Shell for LLM 0.399
MD5 Hash in Rust 0.357
Fix Return Local Reference 0.347