Fix TS Error: useAtom
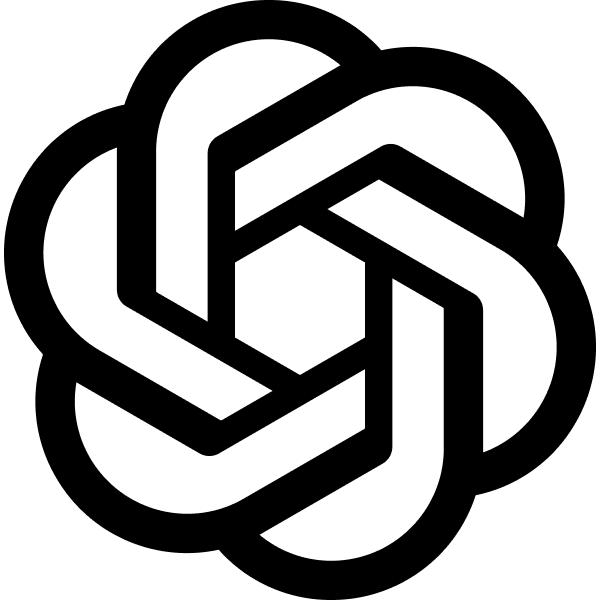
TODO: collapasable, copyable, download, etc
_authMode is a jotai atom with a string representation of my enum.
I can't do _authMode === AuthType.GoogleOAuth
because I get error TS2367: This comparison appears to be unintentional because the types 'PrimitiveAtom<string> & WithInitialValue<string>' and 'AuthType' have no overlap.
How can I fix this?_authMode is a string from a jotai atom with a string representation of my enum.
const [_authMode] = useState(authModeState);
I can't do _authMode === AuthType.GoogleOAuth
because I get error TS2367: This comparison appears to be unintentional because the types 'PrimitiveAtom<string> & WithInitialValue<string>' and 'AuthType' have no overlap.
How can I fix this?The error you're encountering is due to trying to directly compare a jotai atom, _authMode
, which holds a string, with an enum value, AuthType.GoogleOAuth
. In TypeScript, this direct comparison is not valid because _authMode
is an atom object, not the string value it contains.
To fix this issue, you need to access the value inside the jotai atom and then perform the comparison. This is done using jotai's hooks (like useAtom
) if you're within a React component. Here’s how you can approach this:
Access the atom value: You need to access the value contained within
_authMode
atom usinguseAtom
hook in your React component. This hook will subscribe the component to the atom's state, and it will re-render the component whenever the atom's state changes.Compare the extracted value: Once you have the value from the atom, you can then compare this string value to your enum.
Here is an example assuming you're within a React functional component:
import { useAtom } from 'jotai';
import { _authMode } from './whereverYourAtomIsDefined';
import { AuthType } from './yourEnumDefinition';
const MyComponent = () => {
// Accessing the atom value
const [authMode] = useAtom(_authMode);
// Now authMode is a string and can be compared to enum value
if (authMode === AuthType.GoogleOAuth) {
console.log("User is authenticated using Google OAuth");
}
// Render your component
return (
<div>
{/* Render logic depending on authMode */}
Authentication method: {authMode}
</div>
);
};
export default MyComponent;
Here are some key things to consider:
- Reactivity: The
useAtom
hook makes your component reactive to the state of_authMode
. Whenever_authMode
updates, your component will re-render with the new value. - Comparison: Ensure that the string values stored in your atom and your enum are exactly the same, including cases, as string comparisons are case-sensitive.
- Enum Declaration: Make sure that the
AuthType
enum is correctly declared and accessible inThe error you're encountering indicates a type mismatch between the_authMode
variable and theAuthType.GoogleOAuth
enum value. This is primarily due to the use ofuseState
with a Jotai atom, which isn't the standard approach for using atoms in Jotai. Instead ofuseState
, you should use Jotai'suseAtom
hook to manage and access the atom's state.
Here's how you can fix this:
Use
useAtom
instead ofuseState
: To correctly manage the state of a Jotai atom, replaceuseState
withuseAtom
. This will ensure that_authMode
directly references the value of the atom rather than being wrapped in another React state.Ensure proper enum comparison: Once you use
useAtom
,_authMode
will correctly hold a string that you can directly compare with your enum values.
Here's how you can refactor your code:
import { useAtom } from 'jotai';
import { authModeState } from './path/to/your/atoms'; // Adjust the import path accordingly
const AuthType = {
GoogleOAuth: 'GoogleOAuth',
// other authentication types...
};
function MyComponent() {
const [_authMode] = useAtom(authModeState);
if (_authMode === AuthType.GoogleOAuth) {
// Do something specific for Google OAuth
}
// Rest of your component logic...
}
Key Changes:
- Switch from
useState
touseAtom
: This allows you to interact directly with the Jotai atom. - Directly compare
_authMode
withAuthType.GoogleOAuth
: Since_authMode
is now correctly set as a string, you can compare it directly with your enum values.
This approach should resolve the TypeScript error and align your code with Jotai's intended usage patterns for managing atom-based state in React applications.My jotai atom doesn't seem to have the correct state. What am I doing wrong?
I'm using NextJs. Server side code page.tsx
return ( <Main session={session} chatSession={chatSession} authMode={authMode()} /> );
authMode()
correctly returns credentials
a string.
main.tsx
authMode,
}: {
authMode: string;
}): React.ReactElement {
const [_, setAuthMode] = useAtom(authModeState);
// logs credentials
console.log('AOK main.tsx Setting atom to be ', authMode);
setAuthMode(authMode);
// logs google (incorrect)
console.log('AOK afterwards authMode = ', _);
return ( ...```
A deeply nested component
```const [_authMode] = useAtom(authModeState);
// logs google (incorrectly)
console.log("AOK DAISignIn useAtom _authMode = ", _authMode);
Here is the module with the atom
export enum AuthType {
Credentials = "credentials",
GoogleOAuth = "google_oauth",
}
console.log('AOK auth_mode_state defaulting to Google');
export const authModeState = atom<string>(AuthType.GoogleOAuth);```It seems like the issue revolves around the mismanagement or misunderstanding of how Jotai atoms update and maintain their state across component renders and potentially across different server-side and client-side renders in a Next.js application.
### Understanding the Problem
1. **Initial Atom State**: You initialize your `authModeState` atom with the value `AuthType.GoogleOAuth`. This is the default state when the atom is first used anywhere in your application.
2. **Server-Side vs. Client-Side State**: In Next.js, any server-rendered page will be executed again on the client side. If your atom's state is set on the server side, this state isn't automatically hydrated to the client side. Instead, on the client side, the atom's state starts with the initial value (`AuthType.GoogleOAuth`), unless specifically managed.
3. **Asynchronous State Updates**: `setAuthMode(authMode)` is asynchronous. This means that when you log the state immediately after setting it (`console.log('AOK afterwards authMode = ', _)`), it might still log the previous state because the state update hasn't been applied yet.
### Solutions
Here’s how you can approach solving these issues:
#### 1. Client-Side Initialization
Ensure that the atom's state is set based on the prop only on the client side, and ensure it's done once. This can be managed using `useEffect` to set the atom's state when the component mounts:
```jsx
import React, { useEffect } from 'react';
import { useAtom } from 'jotai';
import { authModeState } from './path/to/your/atoms';
export default function Main({ authMode }) {
const [_, setAuthMode] = useAtom(authModeState);
useEffect(() => {
console.log('Setting atom to be ', authMode);
setAuthMode(authMode);
}, [authMode]); // Dependency array to ensure it runs once
return (
// Your component JSX
);
}
This way, you are setting the atom's state only when the component mounts and whenever the authMode
prop changes, ensuring it captures the correct state from server-side rendering.
2. Debugging Asynchronous Updates
To debug state correctly, avoid logging it right after setting it due to the asynchronous nature of state updates:
// Incorrect way to expect updated state immediately after setting it
setAuthMode(authMode);
console.log('After setting state: ', _); // Likely logs old state
// Correct approach: Use useEffect to observe changes
useEffect(() => {
console.log('Updated state: ', _);
}, [_]); // Dependency on the atom's state
3. Ensuring Proper Server-Side Handling
If you need the server-side and client-side states to be consistent, consider initializing your Jotai atom state with a value that can be universally set both server-side and client-side without discrepancies. If the atom needs to retain state across re-renders and navigation, you may want to look into state persistence solutions or ensure the initial value is always derived from props or a context that is reliably populated both server-side and client-side.
By following these steps, you should be able to manage your atom state more effectively in a Next.js environment and resolve the inconsistencies you're experiencing.I updated the code to useEffect. I see in the logging that the nested component is rendered before the useEffect takes place. How can I get that component to re-render ?When dealing with React state updates and component renders, the sequence of updates and renders is important, especially when using hooks like useEffect
that schedule updates asynchronously. Since you're observing that the deeply nested component is rendered before the useEffect
has a chance to update the state in your Main
component, the nested component won't have access to the updated state on its first render.
Making the Nested Component Re-render
Leverage Atom Updates:
- Atoms in Jotai are designed to trigger re-renders in all components that use them when their values change. If the nested component uses
useAtom
to access theauthModeState
, it should automatically re-render whensetAuthMode
is called in theMain
component due to the change in the atom's state. - Make sure that both your
Main
component and the nested component are correctly hooked into the same atom without any scoping issues.
- Atoms in Jotai are designed to trigger re-renders in all components that use them when their values change. If the nested component uses
Use Effect Dependencies Correctly:
- Ensure that the
useEffect
in yourMain
component correctly lists all dependencies to avoid missing updates:useEffect(() => { console.log('Setting atom to be ', authMode); setAuthMode(authMode); }, [authMode, setAuthMode]); // Ensure all dependencies are listed
- This ensures the effect runs anytime
authMode
changes, updating the atom and triggering renders in all subscribed components.
- Ensure that the
Debugging State Propagation:
- You might want to add additional logging inside the effect to ensure that it's being called as expected. Also, confirm that the value of
authMode
received by theMain
component is as expected.
- You might want to add additional logging inside the effect to ensure that it's being called as expected. Also, confirm that the value of
Ensure Proper Propagation:
- Sometimes, due to the asynchronous nature of state updates, there might be a delay in propagation. Ensure your application's structure allows for these updates to propagate through all child components. This can typically be checked by using React Developer Tools to inspect the component tree and the associated states.
Force a Re-render:
- If, for some reason, the nested component does not automatically re-render when the atom updates (which would be unusual with Jotai), you could consider lifting state up or moving some logic into a context that forces a re-render more aggressively. However, this should be unnecessary with correct usage of Jotai.
Example Adjustment
If your nested component still doesn't re-render despite these setups, check how it's consuming the atom. It should look something like this:
import { useAtom } from 'jotai';
import { authModeState } from './path/to/your/atoms';
function NestedComponent() {
const [authMode] = useAtom(authModeState);
console.log("Nested Component authMode: ", authMode);
return (
<div>
Current Authentication Mode: {authMode}
</div>
);
}
Final Check
Make sure there are no errors or unusual behavior in the way atoms are imported or used across different files or components. Sometimes, simple mistakes like importing different instances of the same atom from different files (due to incorrect file paths, for example) can cause such issues.
This setup should ensure that changes in atom state due to updates in your Main
component propagate correctly and trigger re-renders across all components that use the atom, including your nested component.Unsupported multimodal_textThe log sequence in the screenshot you've provided suggests that there's an unexpected behavior happening with the state updates and rendering flow of your components. The nested component (DAISignIn
) is reading the initial state of the atom (google_oauth
) multiple times, even after the Main
component has supposedly set it to credentials
.
Here's a potential sequence of events based on the logs:
- The
authModeState
atom is initialized withgoogle_oauth
. - The
DAISignIn
component renders, accessing the initial state of the atom (google_oauth
). - The
Main
component logs that it's setting the atom tocredentials
. - The
Main
component logs that the atom state is stillgoogle_oauth
immediately after setting it, which is expected due to the asynchronous nature of state updates. - Steps 3 and 4 repeat, indicating the
Main
component might be rendering twice, perhaps once on the server and once on the client, trying to set the atom's state each time. - The
DAISignIn
component logs reading the state (google_oauth
) multiple times, which suggests it's either rendering multiple times before the state is updated or not re-rendering in response to the atom's state change.
Considering these points, here's what you can look into:
Hydration Mismatch:
- Next.js might be re-rendering your components on the client, and state set on the server isn't preserved in the client without hydration. If your
Main
component is setting the atom's state in a server-side render, this state needs to be re-initialized on the client. Next.js doesn't automatically hydrate Jotai atom states.
- Next.js might be re-rendering your components on the client, and state set on the server isn't preserved in the client without hydration. If your
Ensure Proper use of
useEffect
:Make sure
useEffect
is used to set the atom state, not just regular function body execution.useEffect
should run after the component mounts, which ensures the nested components have the chance to access the updated state:useEffect(() => { setAuthMode(authMode); }, [authMode]); // Dependency on authMode prop
Confirm
useEffect
Runs on Client Side:- Ensure that
useEffect
runs on the client side. Since Next.js executes code server-side first, you might set the state on the server whereuseEffect
doesn't run. Then, when the code runs on the client, the state should be set again insideuseEffect
, which does run on the client side.
- Ensure that
Check Atom Imports:
- Double-check that
authModeState
is imported from the same file everywhere it's used to avoid situations where different instances of the atom could be created due to module resolution issues.
- Double-check that
Examine Multiple Renders:
- Investigate why the
Main
component appears to be rendering multiple times and setting the atom's state each time. Is there a prop change causing a re-render, or is it related to the server-client transition in Next.js?
- Investigate why the
Debugging and Tracing:
- Use React Developer Tools to trace when and why components re-render. Check for props or state changes triggering unexpected re-renders.
- You might want to use Jotai's
useAtomDevtools
to track atom changes if you're not already.
Lastly, confirm that you are using the latest stable versions of your dependencies (React, Next.js, Jotai) to ensure there are no bugs or compatibility issues causing unexpected behavior. If you continue to encounter issues, consider reaching out to the Jotai community or reviewing the Jotai documentation for any known issues with SSR (Server-Side Rendering) and state management in Next.js applications.Would adding "use client" to DAISIgnIn.tsx remove the hydration mismatch?The "use client"
directive is a specific experimental feature of Next.js 13 (introduced in late 2022), which allows a component to skip server rendering and render only on the client-side. By using this directive, you indicate that a component should only mount and render in the browser, effectively ensuring that all state management within that component is client-side only.
If you apply "use client"
to DAISignIn
, it will indeed prevent any server-side rendering for that component and may help mitigate hydration mismatches because it guarantees that the component's state is only initialized and managed in the browser. Here's how you can use it:
// DAISignIn.tsx
export default function DAISignIn() {
// This tells Next.js to only use this component on the client side
"use client";
const [authMode] = useAtom(authModeState);
// Rest of your component...
}
However, while this might solve the immediate hydration issue for the DAISignIn
component, it doesn't address the root of the problem if the state needs to be consistent between the server and the client or across multiple components. It also means that DAISignIn
will not be rendered during server-side rendering or static generation, which could affect performance and SEO for your page.
Here are a few things to keep in mind when using "use client"
:
- SEO: Client-only components are not visible to search engine crawlers, which could negatively impact the SEO of your page.
- Performance: The component will only be rendered after the JavaScript is downloaded and executed in the browser, which might lead to a visible delay in rendering the component (commonly referred to as a flash of unstyled content, or FOUC).
- State Management: This approach only addresses the symptoms of state mismanagement, not the cause. It's often better to ensure that the state is managed consistently across server and client.
Before using "use client"
, consider whether there are other ways to resolve the issue that maintain server-side rendering capabilities, such as ensuring proper state hydration or managing state so that it's consistent between server and client. If the authMode
state is critical for the initial render and SEO, you might want to use Next.js data fetching methods like getServerSideProps
to fetch and pass down the necessary state as props, and then initialize the Jotai atom with that state on the client side.Doesn't "use client"; go at the top of the JS file and not in the function?Yes, you are correct. The use client
directive should be at the top of the file in Next.js 13 and later versions. It's a top-level directive that tells Next.js to only render the component on the client-side, skipping the server rendering altogether. Here's how it should be used:
// DAISignIn.tsx
"use client";
export default function DAISignIn() {
const [authMode] = useAtom(authModeState);
// Rest of your component...
}
Placing "use client"
at the top of the file outside of any functions or other logic ensures that it's executed as part of the module evaluation and that Next.js correctly interprets it as a directive for client-side-only rendering. This way, it informs the framework about the rendering behavior before any component logic is run.Looking at my NodeJS cli logs, I still see AOK DAISignIn useAtom _authMode = google_oauth, so the component must be still rendering server sideIf you're seeing logs from DAISignIn
on your Node.js CLI, then the component is indeed still being rendered on the server side. This can happen for a few reasons:
Next.js Version: The
use client
directive is a feature of Next.js 13, which is experimental. If you're not using Next.js 13 or if the feature isn't stable in your version, it might not work as expected.Correct Usage: The
use client
directive must be the very first line in your file, before any imports or other code.Experimental Features: Since
use client
is an experimental feature, it's important to ensure that you have enabled the correct experimental flags in yournext.config.js
if required.Misconfiguration: There might be a configuration issue within your Next.js setup that's causing the directive to be ignored.
Nested Components: If
DAISignIn
is being rendered within another component that's server-side rendered, that could be another reason why you're seeing server-side logs.
To debug this issue:
- Make sure you are using the latest version of Next.js 13.
- Ensure that your
next.config.js
is properly configured to use the experimental features of Next.js 13. - Verify that
use client
is the very first line of code in yourDAISignIn
component file, with no code or comments before it. - If you are conditionally rendering
DAISignIn
within another component, make sure the condition is based on client-side-only variables, such as state that is only set after hydration.
If after checking all the above points, you're still facing the issue, consider the following:
Client-side Rendering: As an alternative to using
use client
, you can conditionally render the component in the client-side code only. This is a more traditional approach prior to theuse client
directive:// In your component file import { useEffect, useState } from 'react'; function DAISignIn() { const [isClient, setIsClient] = useState(false); const [authMode] = useAtom(authModeState); useEffect(() => { // Once the component mounts, this will be true setIsClient(true); }, []); if (!isClient) { return null; // Or a loader, etc. } // Rest of your component... }
This
useState
anduseEffect
pattern ensures that your component only renders on the client side.Server/Client Consistency: If the state consistency between server and client is crucial for your application, you may need to ensure that your initial state is serialized and passed from the server to the client correctly.
Review Logs: If you're using a custom server or a complicated setup, ensure that the logs you're seeing are actually coming from server-side rendering and not from an earlier build process or a different part of the application.
Lastly, if you're still unable to resolve the issue, you may want to consider reaching out to the Next.js community or reviewing the Next.js documentation and GitHub issues for any similar reports or solutions regarding the use client
directive.
Related Chats
Fix TS Error: useAtom 1.000
React Auth Types Enum 0.519
Rust Enum for Strings 0.438
Scraper Implementation with Enum 0.386
Provider vs useAtom: when? 0.384
Teste de Autenticação Vitest 0.381
Atom Access Methods 0.362
React TypeScript - Handle Unknown 0.339
TS Default Value Fix 0.339
Force Sync Read Atom. 0.324